In as we speak’s tutorial, we’ll present you the way to listing all of the cash in an ETH deal with utilizing the Moralis Token API – the {industry}’s main device for ERC-20 knowledge. Due to the accessibility of the Token API, you will get all of the cash of any deal with with a single name to the getWalletTokenBalances() endpoint. Right here’s just a little sneak peek of what the code would possibly seem like:
const Moralis = require(“moralis”).default;
const { EvmChain } = require(“@moralisweb3/common-evm-utils”);
const runApp = async () => {
await Moralis.begin({
apiKey: “YOUR_API_KEY”,
// …and some other configuration
});
const deal with = “0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d”;
const chain = EvmChain.ETHEREUM;
const response = await Moralis.EvmApi.token.getWalletTokenBalances({
deal with,
chain,
});
console.log(response.toJSON());
};
runApp();
You merely have so as to add your Moralis API key, configure deal with to suit your question and run the code. In return, you’ll get a response containing a listing of all of the cash from the desired ETH deal with.
That’s it; it doesn’t should be tougher than that when working with Moralis. Nonetheless, if you’d like a extra detailed breakdown of your entire course of, be a part of us on this tutorial or take a look at the Moralis documentation web page on the way to get all tokens owned by an deal with!
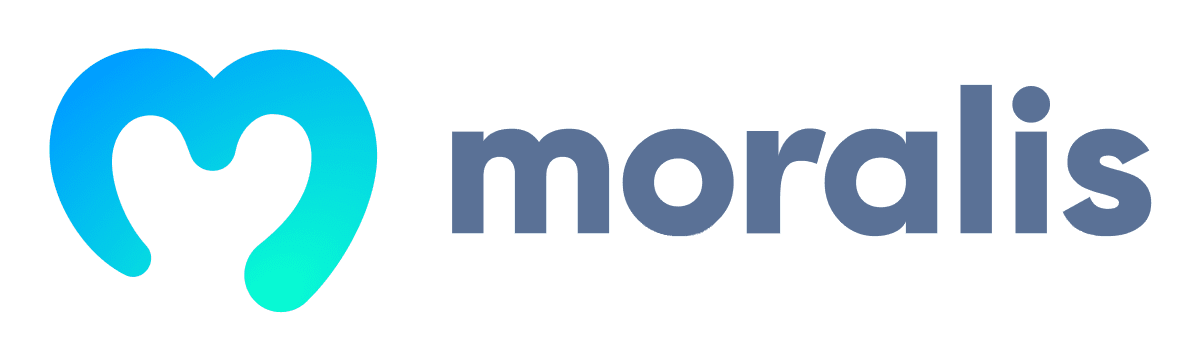
Additionally, should you want to name the Token API your self, don’t neglect to enroll with Moralis. You’ll be able to arrange your account with out paying a dime, and also you’ll get on the spot entry to all our industry-leading Web3 APIs!
Overview
We’ll kickstart as we speak’s article by diving into the ins and outs of ETH addresses. In doing so, we’ll cowl what they’re and what they’re used for. From there, we’ll soar straight into the tutorial and present you the way to listing all of the cash in an ETH deal with in three easy steps utilizing Moralis’ Token API:
Get a Moralis API KeyWrite a Script Calling the getWalletTokenBalances() EndpointRun the Code
From there, we’ll discover some outstanding use instances for itemizing all of the cash in an ETH deal with earlier than we high issues off by diving deeper into Moralis!
Now, with out additional ado, let’s get going and discover the intricacies of ETH addresses!
What’s an ETH Deal with?
An ETH deal with is a 42-character lengthy, alphanumerical hex string serving as a singular and public identifier for an externally owned account (EOA) or good contract on the Ethereum blockchain. Such an deal with is usually used for sending, receiving, and storing digital belongings like fungible and non-fungible tokens (NFTs)!
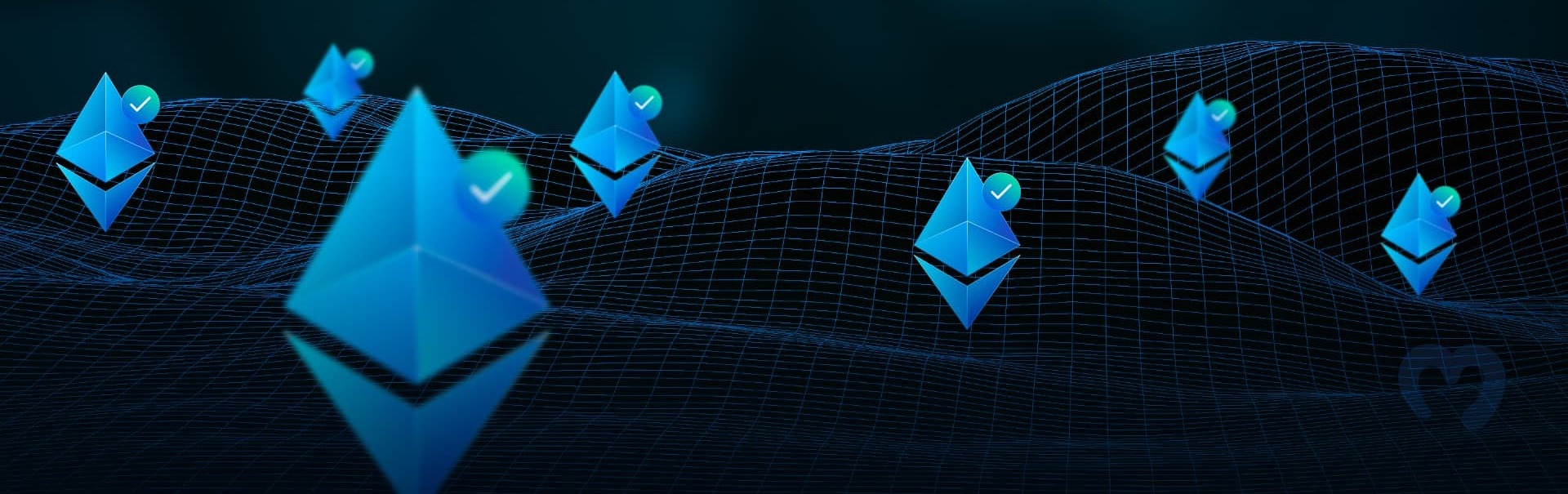
There are two forms of ETH addresses, and we’ll briefly cowl each under:
Account Addresses: An account deal with – additionally generally known as a ”pockets deal with” or ”blockchain deal with” – corresponds to a public consumer account on the blockchain managed by a personal key. Account addresses are used to ship, obtain, and retailer digital belongings, together with interacting with good contracts and dapps. Contract Addresses: Contract addresses are related to good contracts deployed on the blockchain. A contract deal with isn’t managed by a person or entity like an account deal with however quite represents the situation the place the good contract resides on the community.
All in all, ETH addresses are distinctive identifiers for Ethereum accounts and good contracts. Moreover, they’re generally used for sending, receiving, and storing digital belongings like fungible tokens and NFTs!
Since ETH addresses are public, we will use them to lookup details about accounts and good contracts. This contains issues comparable to token balances, which we’ll present you how one can fetch within the following part utilizing Moralis!
Full Tutorial: Record All of the Cash in An ETH Deal with
The simplest option to listing all of the cash in an ETH deal with is to leverage Moralis’ Token API – the final word interface for real-time ERC-20 knowledge. In reality, because of the accessibility of this industry-leading device, you will get all the data you want straight from the Ethereum blockchain in three easy steps:
Get a Moralis API KeyWrite a Script Calling the getWalletTokenBalances() EndpointRun the Code
Nonetheless, earlier than leaping into the preliminary step of this tutorial, you will need to first handle a few conditions!
Conditions
Moralis’ Web3 APIs are appropriate with a number of programming languages, together with Python, TypeScript, and so on. Nonetheless, for this tutorial, we’ll be sticking to JavaScript. As such, earlier than you proceed, please ensure you have the next prepared:
Step 1: Get a Moralis API Key
To name the Token API, you want a Moralis API key, which you will get without cost by signing up with Moralis. As such, should you haven’t already, click on on the ”Begin for Free” button on the high proper of the Moralis homepage to create your account:
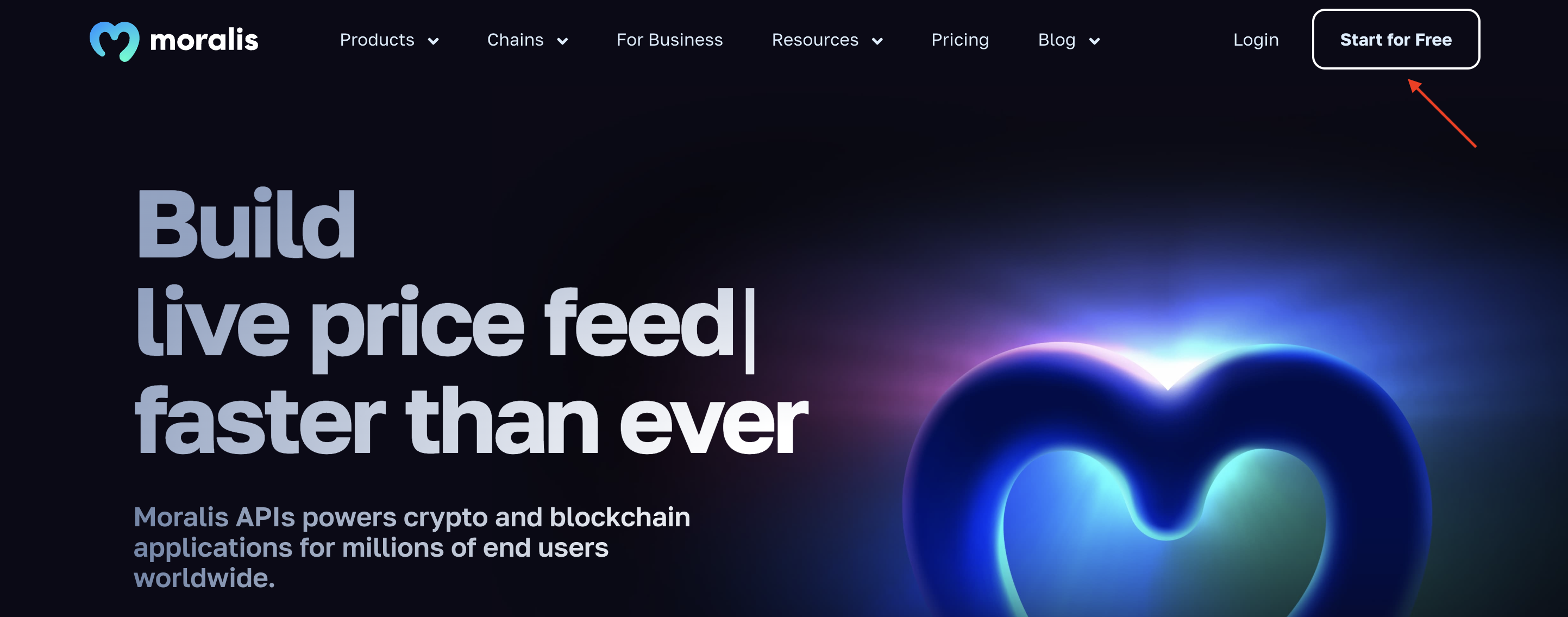
With an account at hand, choose a mission, go to the ”Settings” tab utilizing the navigation bar to the left, scroll right down to the ”API Keys” part, and duplicate your key:
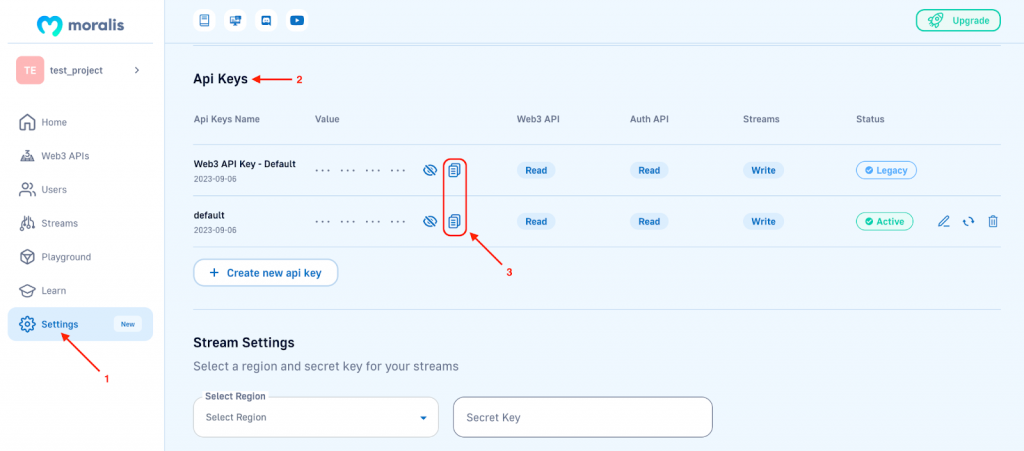
Save the important thing for now, as you’ll want it within the subsequent step when initializing the Moralis software program growth equipment (SDK)!
Step 2: Write a Script Calling the getWalletTokenBalances() Endpoint
For the second step, begin by organising a brand new mission folder in your most popular IDE. From there, open a brand new terminal and run the next command within the root folder to put in the Moralis SDK:
npm set up moralis @moralisweb3/common-evm-utils
Subsequent, create a brand new ”index.js” file and add the next code:
const Moralis = require(“moralis”).default;
const { EvmChain } = require(“@moralisweb3/common-evm-utils”);
const runApp = async () => {
await Moralis.begin({
apiKey: “YOUR_API_KEY”,
// …and some other configuration
});
const deal with = “0xbc4ca0eda7647a8ab7c2061c2e118a18a936f13d”;
const chain = EvmChain.ETHEREUM;
const response = await Moralis.EvmApi.token.getWalletTokenBalances({
deal with,
chain,
});
console.log(response.toJSON());
};
runApp();
Earlier than working the code, you should make a couple of minor configurations. Begin by changing YOUR_API_KEY with the important thing you copied throughout step one. Doing so will initialize the Moralis SDK:
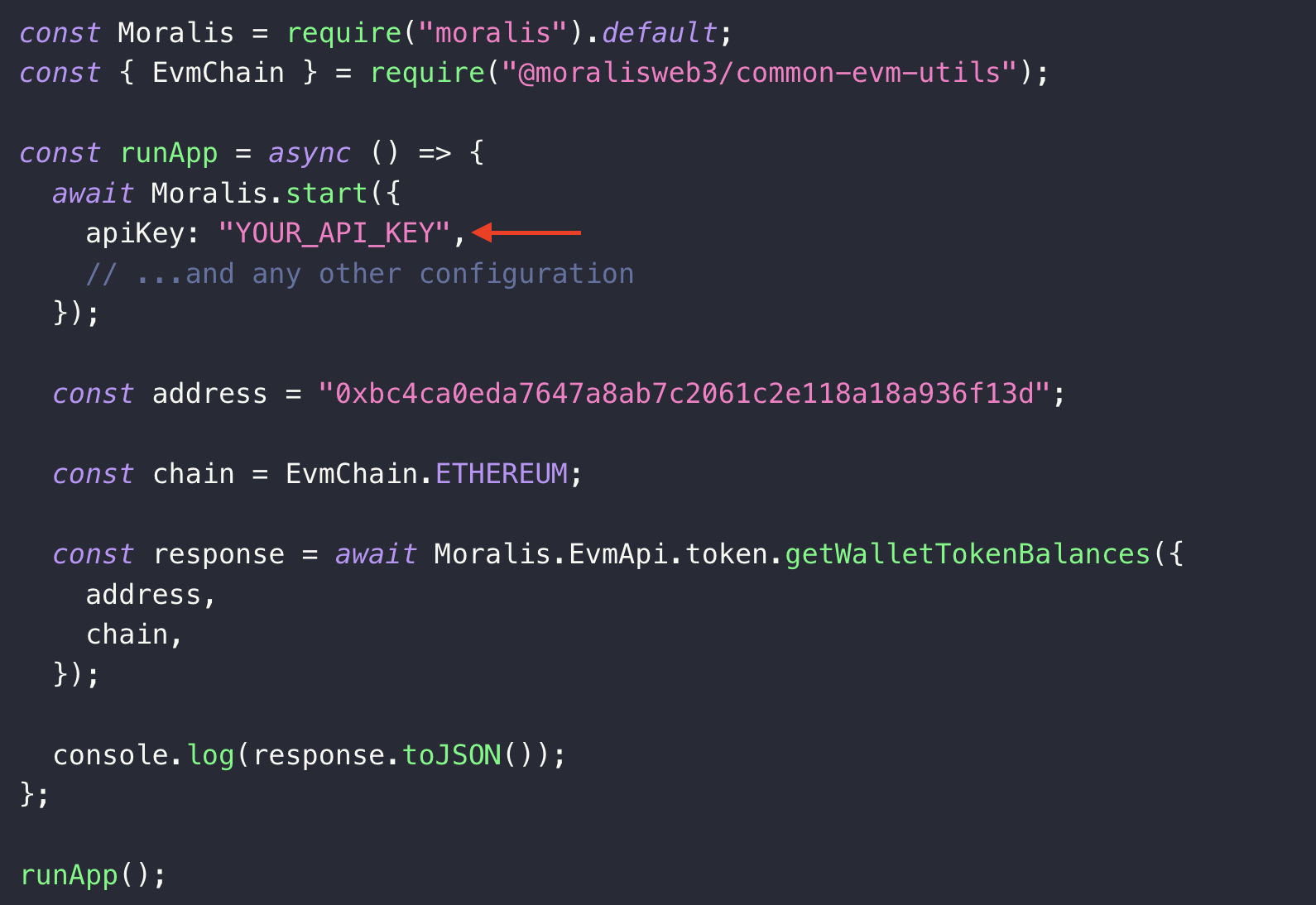
Understand that you don’t wish to expose your Moralis API key. As such, when constructing production-ready dapps, be certain that to make use of surroundings variables and different finest practices.
From right here, you additionally must configure the deal with const by including the ETH deal with from which you wish to get the listing of all cash:
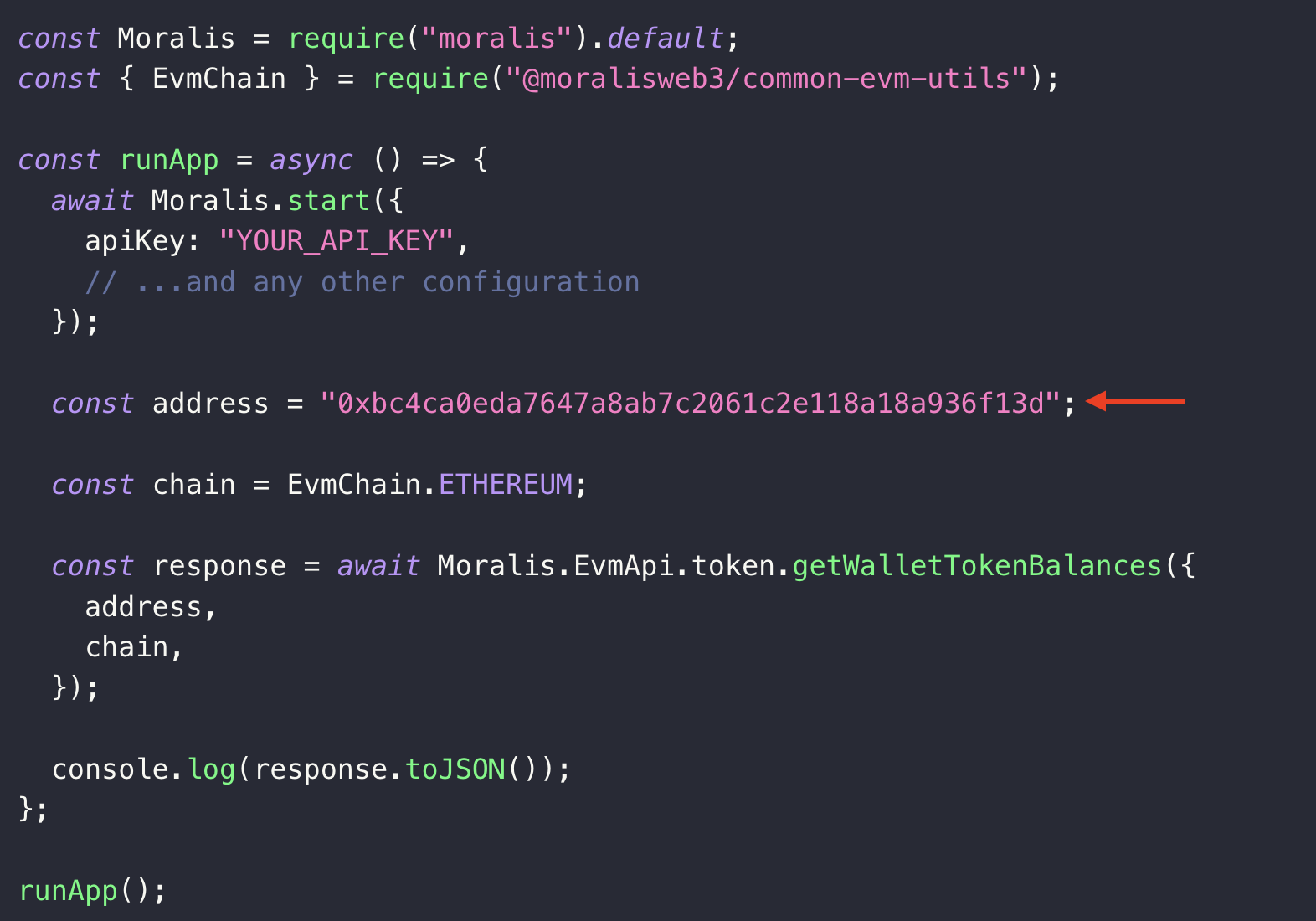
We then move alongside deal with and chain as parameters when calling the getWalletTokenBalances() endpoint:
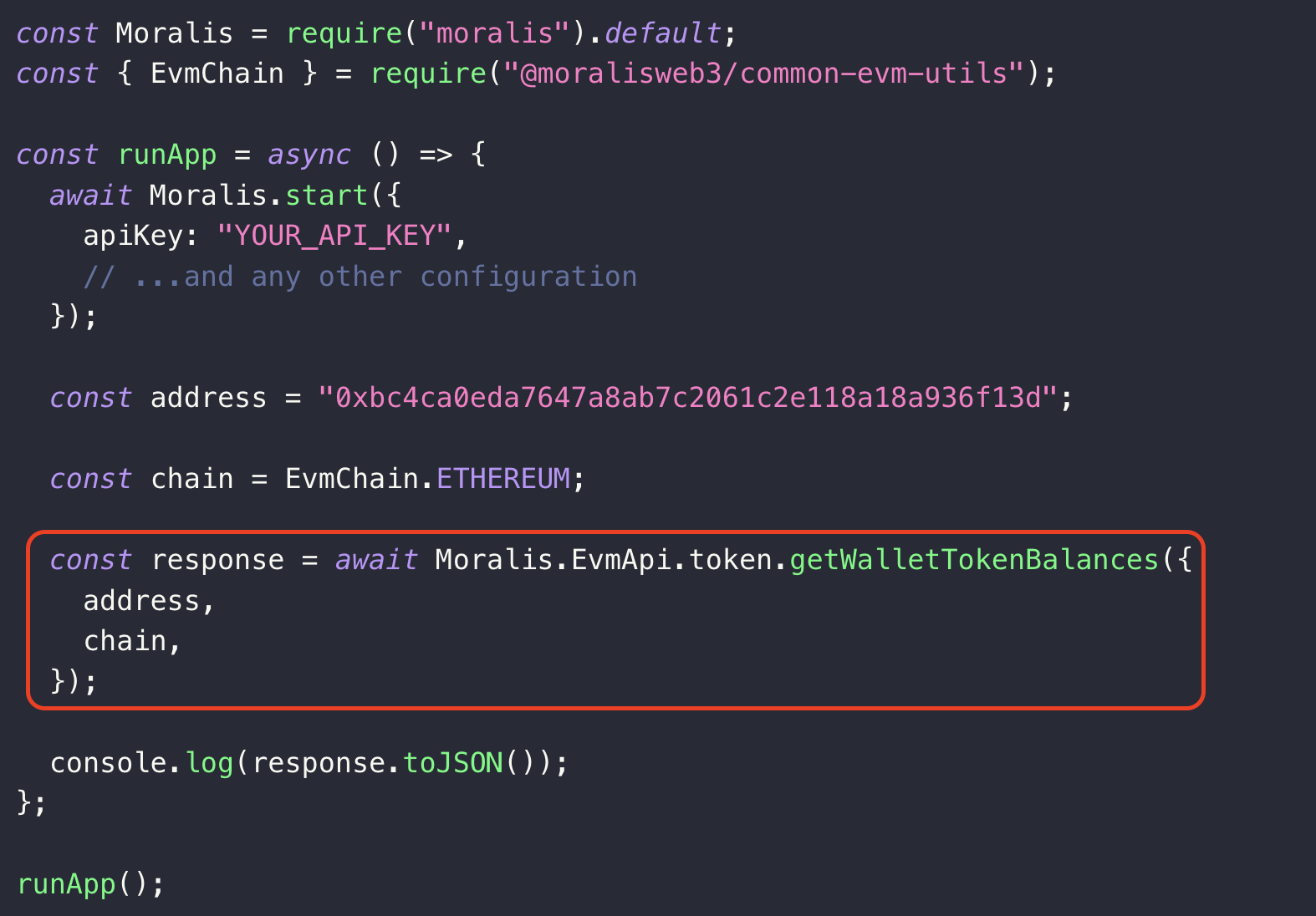
That’s it for the code; you’re now able to run the script and fetch a listing of all of the cash within the specified ETH deal with!
Step 3: Run the Code
To run the code, go forward and launch a brand new terminal, cd into the mission’s root folder, and execute the next command:
node index.js
When you run the code, you’ll get a response containing a listing of all of the cash within the specified ETH deal with. It ought to look one thing like this:
[
{
“token_address”: “0xff20548405c6f024264b5c8dc954625285b799e1”,
“symbol”: “BTCETF”,
“name”: “BTCETF”,
“logo”: null,
“thumbnail”: null,
“decimals”: 18,
“balance”: “166000000000000000000000”,
“possible_spam”: false
},
{
“token_address”: “0xe90cc7d807712b2b41632f3900c8bd19bdc502b1”,
“symbol”: “KUMA”,
“name”: “Kuma”,
“logo”: null,
“thumbnail”: null,
“decimals”: 18,
“balance”: “3000000000000000000000000000”,
“possible_spam”: false
},
//…
]
Congratulations; you now know the way to get a listing of all of the cash in an ETH deal with!
Now, if you’d like inspiration on what to do with this knowledge, be a part of us within the following part. In doing so, we’ll discover some frequent use instances for itemizing all of the cash in an ETH deal with!
Use Instances for Itemizing All of the Cash in An ETH Deal with
There are lots of use instances for itemizing all of the cash in an ETH deal with, and we gained’t be capable of cowl all of them on this part. As a substitute, we’ll briefly listing 4 outstanding examples of platforms that want entry to deal with balances in some capability:
Portfolio Trackers: A crypto portfolio tracker is a digital platform that means that you can oversee all of your cryptocurrency holdings in a single place. Some nice examples embrace Delta, Koinly, and Kubera. Decentralized Exchanges (DEXs): A DEX is a crypto trade facilitating peer-to-peer (P2P) transactions with out middleman involvement. Examples of well-established platforms embrace Uniswap, Curve Finance, and PancakeSwap. Block Explorers: A block explorer is a digital device that means that you can seek for real-time and historic details about a blockchain community. This contains every thing from block knowledge to token balances of a specific deal with. Some outstanding examples of block explorers embrace Etherscan, PolygonScan, and BscScan. Web3 Wallets: Web3 wallets are digital platforms that mean you can retailer and handle your digital belongings, together with shopping for, promoting, and swapping tokens. Examples of Web3 wallets are MetaMask, Coinbase Pockets, and Ledger.
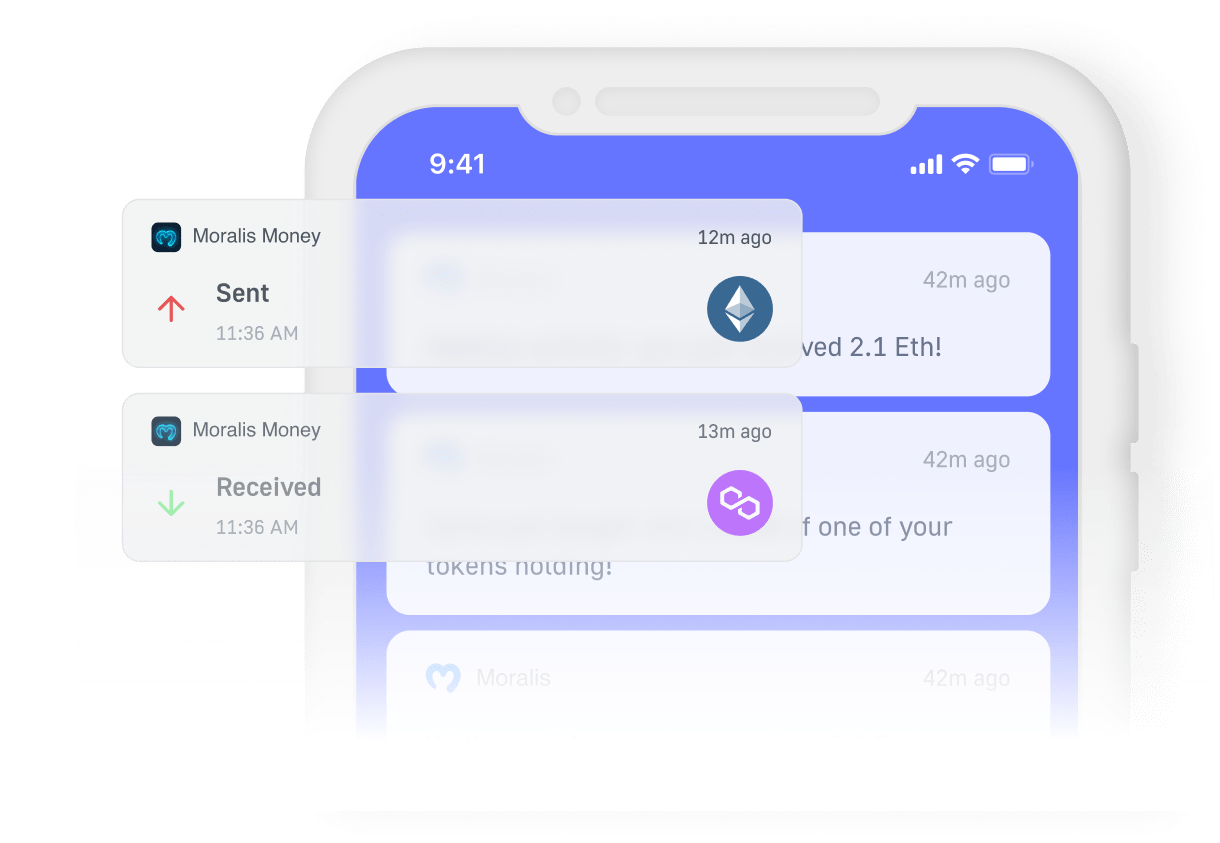
Now that you understand how to listing all of the cash in an ETH deal with and a few situations when this knowledge might be useful, let’s discover Moralis’ capabilities additional within the subsequent part!
Past Querying a Record of All of the Cash in An ETH Deal with – Exploring Moralis Additional
Moralis is the {industry}’s premier Web3 API supplier, and the capabilities of our dynamic toolset prolong far past the Token API!
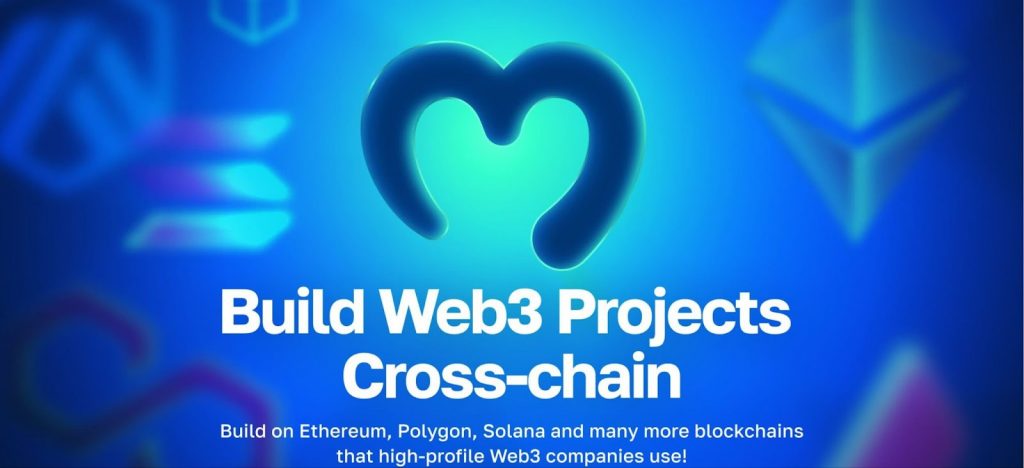
In Moralis’ industry-leading Web3 API suite, you’ll discover a number of interfaces for varied use instances, and down under, we’ll discover three examples:
Pockets API: Our Pockets API is the final word device should you’re trying to construct Web3 wallets or combine pockets performance into your dapps. With single API calls, you possibly can effortlessly fetch pockets balances, real-time transfers, profile knowledge, and far more. As an example, right here’s an instance of how straightforward it’s to fetch the native stability of any pockets with the getNativeBalance() endpoint: const response = await Moralis.EvmApi.stability.getNativeBalance({
“chain”: “0x1”,
“deal with”: “0xDC24316b9AE028F1497c275EB9192a3Ea0f67022”
}); NFT API: Moralis’ NFT API is the {industry}’s main device for NFT-related knowledge. With this premier interface, you will get NFT metadata, balances, on-chain costs, and extra with solely single strains of code. For instance, with the getWalletNFTs() endpoint, you possibly can seamlessly fetch the NFT stability of any pockets: const response = await Moralis.EvmApi.nft.getWalletNFTs({
“chain”: “0x1”,
“deal with”: “0xff3879b8a363aed92a6eaba8f61f1a96a9ec3c1e”
}); Blockchain API: With the Blockchain API, you possibly can unlock the ability of uncooked and decoded blockchain knowledge. Fetch block knowledge, good contract logs, occasions, and far more with ease. As an example, right here’s an instance of how one can get block knowledge – together with hashes, timestamps, and so on. – with the getBlock() endpoint: const response = await Moralis.EvmApi.block.getBlock({
“chain”: “0x1”,
“blockNumberOrHash”: “18541416”
});
So, it doesn’t matter should you’re constructing Web3 wallets, NFT dapps, blockchain explorers, or some other platform; Moralis has the right instruments in your wants. If you happen to’d prefer to discover all our premier interfaces, take a look at our official Web3 API web page!
Nonetheless, at this level, you would possibly nonetheless be asking your self, ”Why ought to I leverage Moralis’ Web3 APIs?”. To reply this query, let’s discover some outstanding advantages of Moralis within the following subsection!
Advantages of Moralis’ Web3 APIs
Apart from permitting you to construct Web3 initiatives quicker and extra effectively, Moralis gives many benefits. Nonetheless, sadly, we will be unable to cowl all the advantages on this article. As a substitute, we’ve narrowed it down to a few outstanding examples of why you need to use Moralis:
High Efficiency: There are lots of good Web3 API suppliers in the marketplace; nevertheless, Moralis stands out as the very best in relation to efficiency. Whether or not you wish to measure by reliability, pace, protection, or some other metric, Moralis at all times comes out on high. Unparalleled Scalability: Moralis’ APIs supply unparalleled scalability. Consequently, our APIs will scale with out fault as your consumer base grows. Cross-Chain Compatibility: Our Web3 APIs are chain agnostic, supporting all main blockchain networks. This contains Ethereum, BNB Good Chain, Solana, and so on., together with a number of outstanding ETH layer-2 scaling options. As such, when working with Moralis, you’ll haven’t any bother constructing cross-chain appropriate dapps!
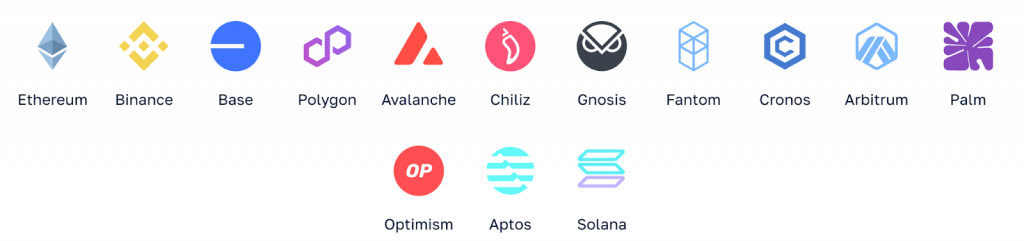
So, should you’re severe about constructing Web3 initiatives, be certain that to enroll with Moralis. You’ll be able to create your account without cost, and also you’ll achieve on the spot entry to all our industry-leading Web3 growth instruments!
Abstract: The right way to Record All of the Cash in An ETH Deal with
In as we speak’s article, we kicked issues off by diving into the ins and outs of ETH addresses. In doing so, we realized that they function distinctive identifiers for EOAs and good contracts on the Ethereum blockchain.
From there, we then jumped straight into the primary tutorial and confirmed you the way to listing all of the cash in an ETH deal with in three steps utilizing the Moralis Token API:
Get a Moralis API KeyWrite a Script Calling the getWalletTokenBalances() EndpointRun the Code
So, when you’ve got adopted alongside this far, you now know the way to fetch all of the tokens of Ethereum addresses!
We then additionally explored some outstanding use instances for this knowledge. In doing so, we briefly coated Web3 wallets, portfolio trackers, DEXs, and block explorers. If you happen to appreciated this tutorial on the way to listing all of the cash in an ETH deal with, take into account studying extra content material right here on the Moralis weblog. For instance, take a look at our most up-to-date Starknet faucet information or discover the ins and outs of Web3 social media.
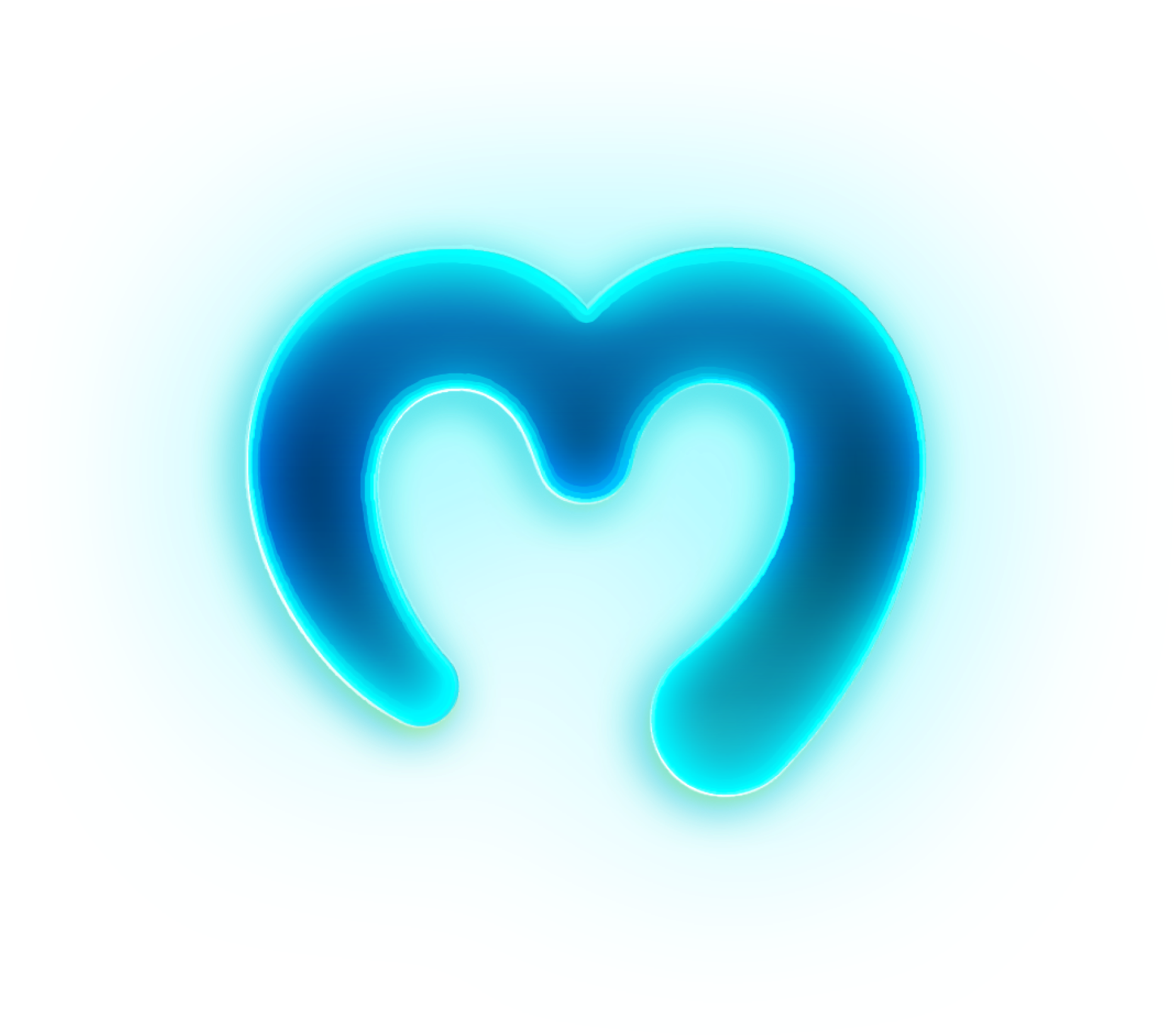
Additionally, if you wish to leverage our Web3 APIs in your growth endeavors, don’t neglect to enroll with Moralis. You’ll be able to arrange your account without cost, and also you’ll be capable of begin constructing dapps quicker and smarter as we speak!