On this article, we’ll present you the right way to create a token on BNB Good Chain (BSC) utilizing Remix, breaking down the method into 5 simple steps. However when you’re wanting to dive straight into the code of our token contract, yow will discover it right here:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import “@openzeppelin/contracts/token/ERC20/ERC20.sol”;
contract BEP20 is ERC20 {
constructor(uint256 initialSupply) ERC20(“BEP20Test”, “BPT”) {
_mint(msg.sender, initialSupply);
}
}
For a extra complete breakdown of the code above, together with detailed directions on compiling and deploying the contract utilizing Remix, we invite you to hitch us on this information, providing a extra in-depth tutorial on the right way to create a token on BSC!
Overview
We’ll kickstart at present’s article by diving into the ins and outs of BSC tokens. From there, we’ll discover Remix IDE and MetaMask, as we’ll use each these instruments to put in writing and deploy our BSC token contract. Subsequent, we’ll soar straight into our tutorial to indicate you the right way to create a BSC token with Remix in 5 straightforward steps:
Add the BSC Testnet to MetaMaskGet BSC Testnet TokensCreate Your BSC Token Contract with RemixDeploy Your ContractAdd the Token to MetaMask
Lastly, for these additional excited about Web3 growth, we’ll additionally introduce you to Moralis – the {industry}’s main Web3 API supplier!
In Moralis’ suite of Web3 APIs, you’ll discover a number of interfaces for varied use circumstances. Some outstanding examples embody the NFT API, Pockets API, and lots of others. With these instruments, you may seamlessly question and combine on-chain information and blockchain performance into all initiatives with just a few traces of code, making Web3 growth a breeze!
Additionally, do you know you may join with Moralis without cost? As such, please take this chance to supercharge your Web3 growth endeavors with our premier Web3 APIs!
Nonetheless, let’s begin this text by answering the query, ”What’s a BSC token?”
What’s a BSC Token?
A BSC token is a digital asset constructed on BNB Good Chain (BSC) – an EVM-compatible blockchain community designed for operating sensible contract-based purposes. Furthermore, BSC tokens adhere to the BEP-20 token commonplace, which defines a algorithm and pointers for creating and managing fungible tokens on the community!
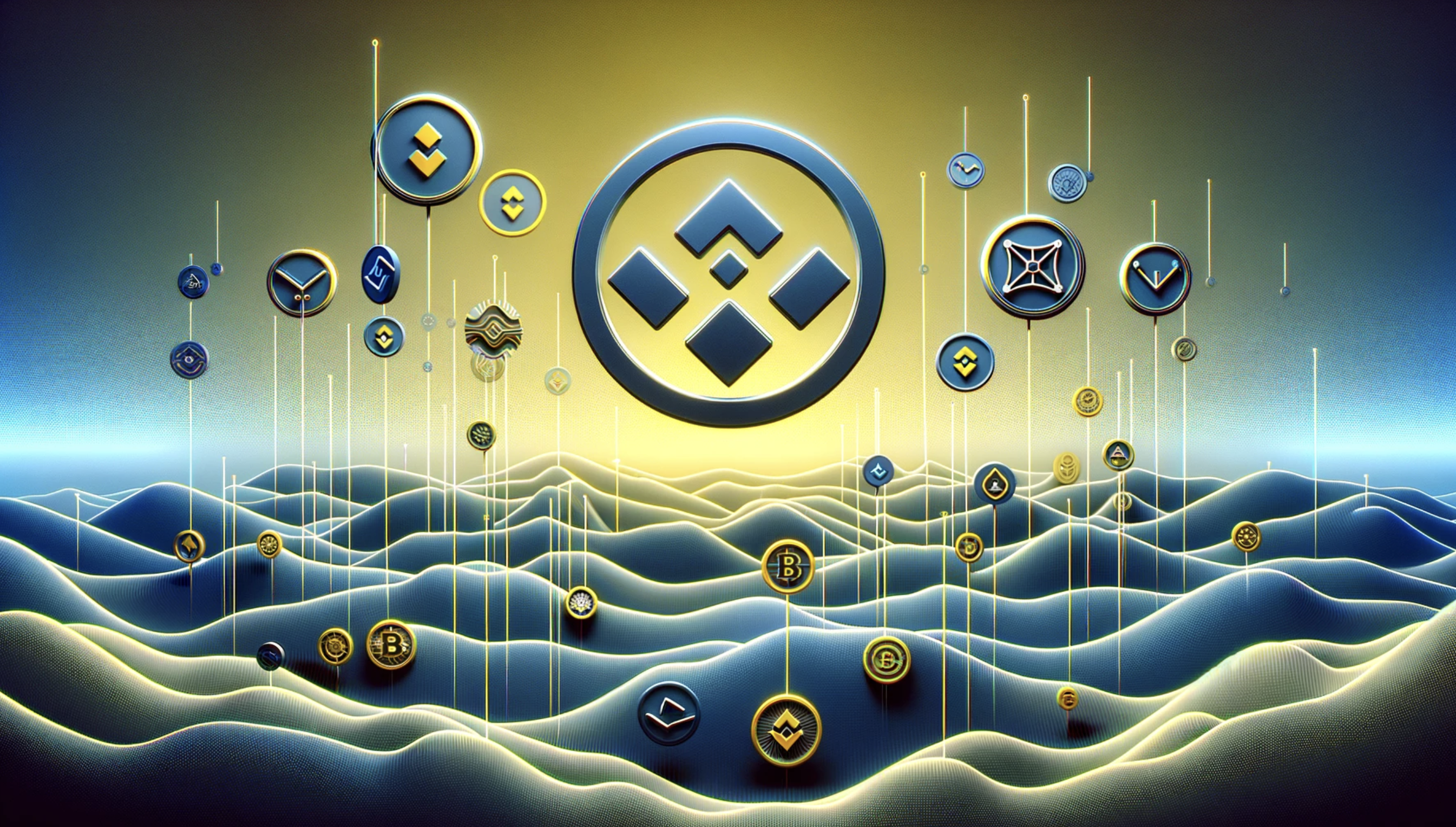
BEP-20 is an extension of Ethereum’s extensively adopted ERC-20 commonplace, and they’re very related. You’ll be able to consider the usual as a blueprint that defines particular features and variables token contracts should implement to be thought of BEP-20 compliant.
By offering a standard commonplace, BEP-20 ensures consistency and interoperability amongst tokens, streamlining the method for builders to work with and combine these digital belongings into their Web3 wallets, decentralized exchanges (DEXs), and different platforms.
In essence, BSC tokens are tokens constructed on BNB Good Chain adhering to the BEP-20 token commonplace!
Making a Token on BSC with Remix & MetaMask
In at present’s article, we’ll present you the right way to create a BSC token utilizing Remix and MetaMask. Consequently, earlier than leaping into the tutorial, let’s briefly break down each these platforms individually:
Remix IDE: Remix is an built-in growth setting (IDE) that includes an intuitive graphical consumer interface and a strong toolset for writing, testing, debugging, compiling, and deploying EVM-compatible sensible contracts. What’s extra, Remix gives a variety of plugins and extensions, permitting you to seamlessly tailor your growth setting to your wants.
All in all, Remix is a robust, intuitive, and well-used platform that has change into a vital device in Web3’s growth ecosystem!
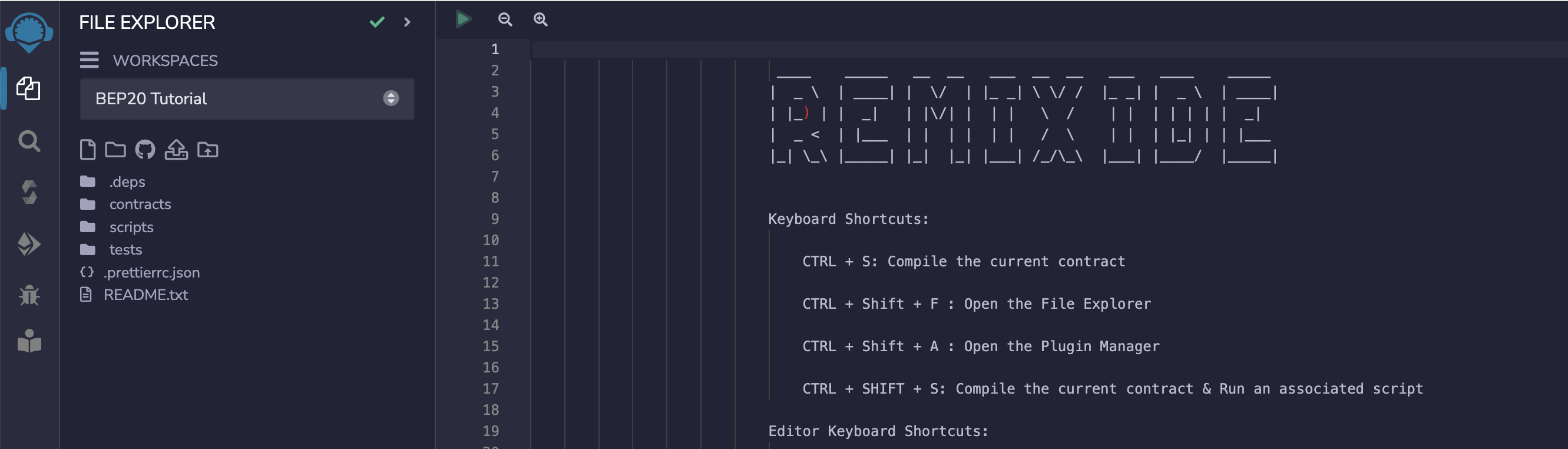
MetaMask: MetaMask is the {industry}’s main Web3 pockets, boasting a formidable 30 million month-to-month energetic customers. With this EVM-compatible pockets, it’s doable to retailer, purchase, ship, and swap tokens throughout a number of chains, together with Ethereum, BSC, Polygon, and lots of others.
Furthermore, along with permitting you to retailer and handle your digital belongings, MetaMask additionally acts as a gateway to Web3’s intensive ecosystem of decentralized purposes (dapps) and sensible contracts!
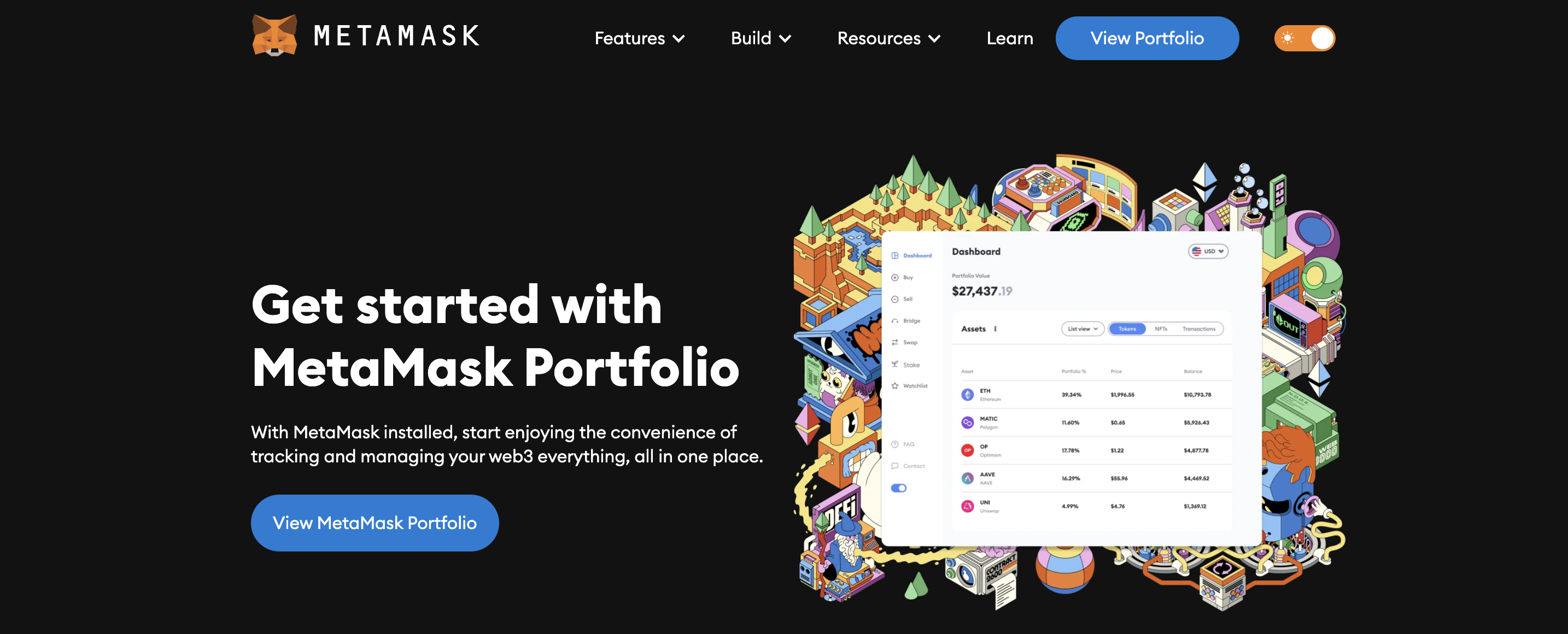
Now, with an outline of Remix and MetaMask, let’s dive straight into the primary tutorial and present you the right way to create a token on BSC!
The best way to Create a BSC Token with Remix
Within the following subsections, we’ll present you the right way to create a BSC token with Remix and MetaMask in 5 steps:
Add the BSC Testnet To MetaMaskGet BSC Testnet TokensCreate Your BSC Token Contract with RemixDeploy Your ContractAdd the Token To MetaMask
On this tutorial, we’ll make the most of the BSC testnet for comfort. Nevertheless, the process for making a token on the BSC mainnet stays practically an identical, with the primary distinctions rising within the first and second steps, the place you have to add the mainnet and get actual BNB tokens as an alternative.
Nonetheless, with out additional delay, let’s soar into step one and present you the right way to add the BSC testnet to MetaMask!
Step 1: Add the BSC Testnet To MetaMask
Including the BSC testnet to MetaMask is easy, and the very first thing you have to do is click on the networks drop-down menu on the prime left of your MetaMask interface, adopted by ”Add community”:
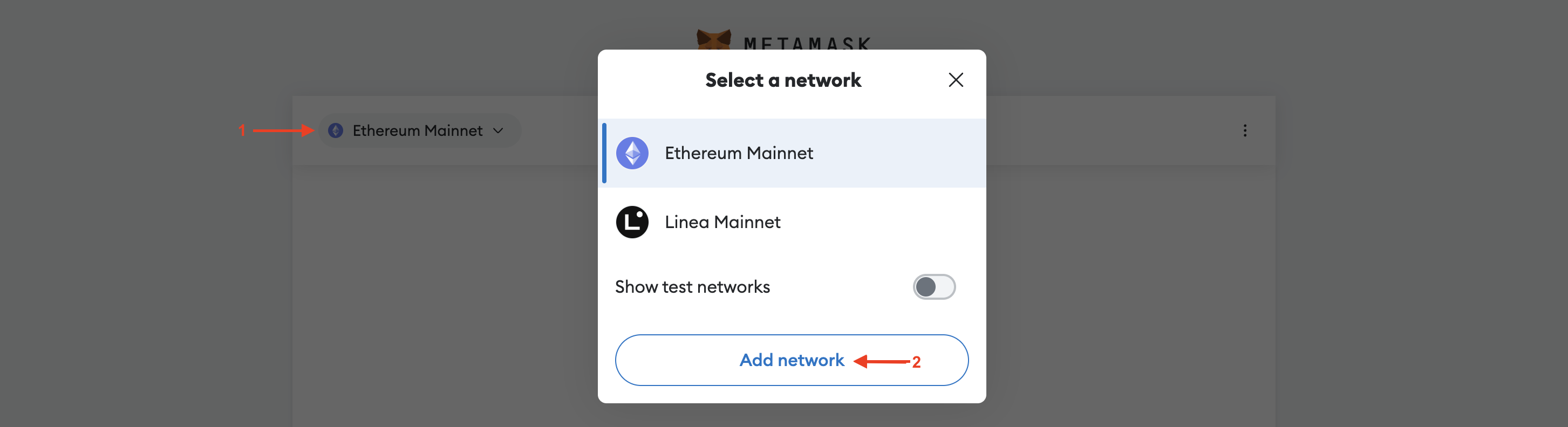
Subsequent, click on ”Add a community manually” on the backside:
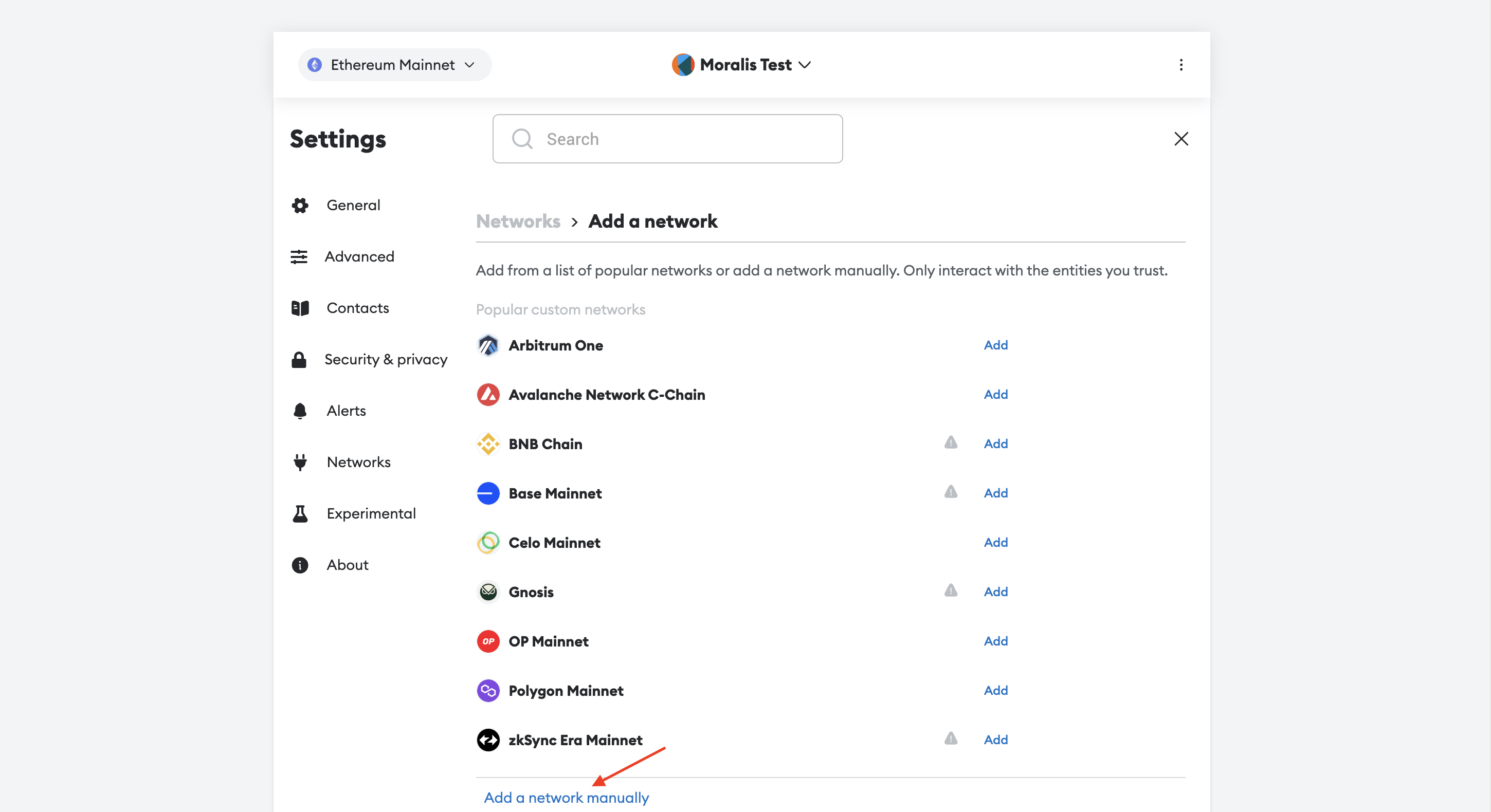
Doing so takes you to the next web page, the place you have to add the community particulars for the BSC testnet:
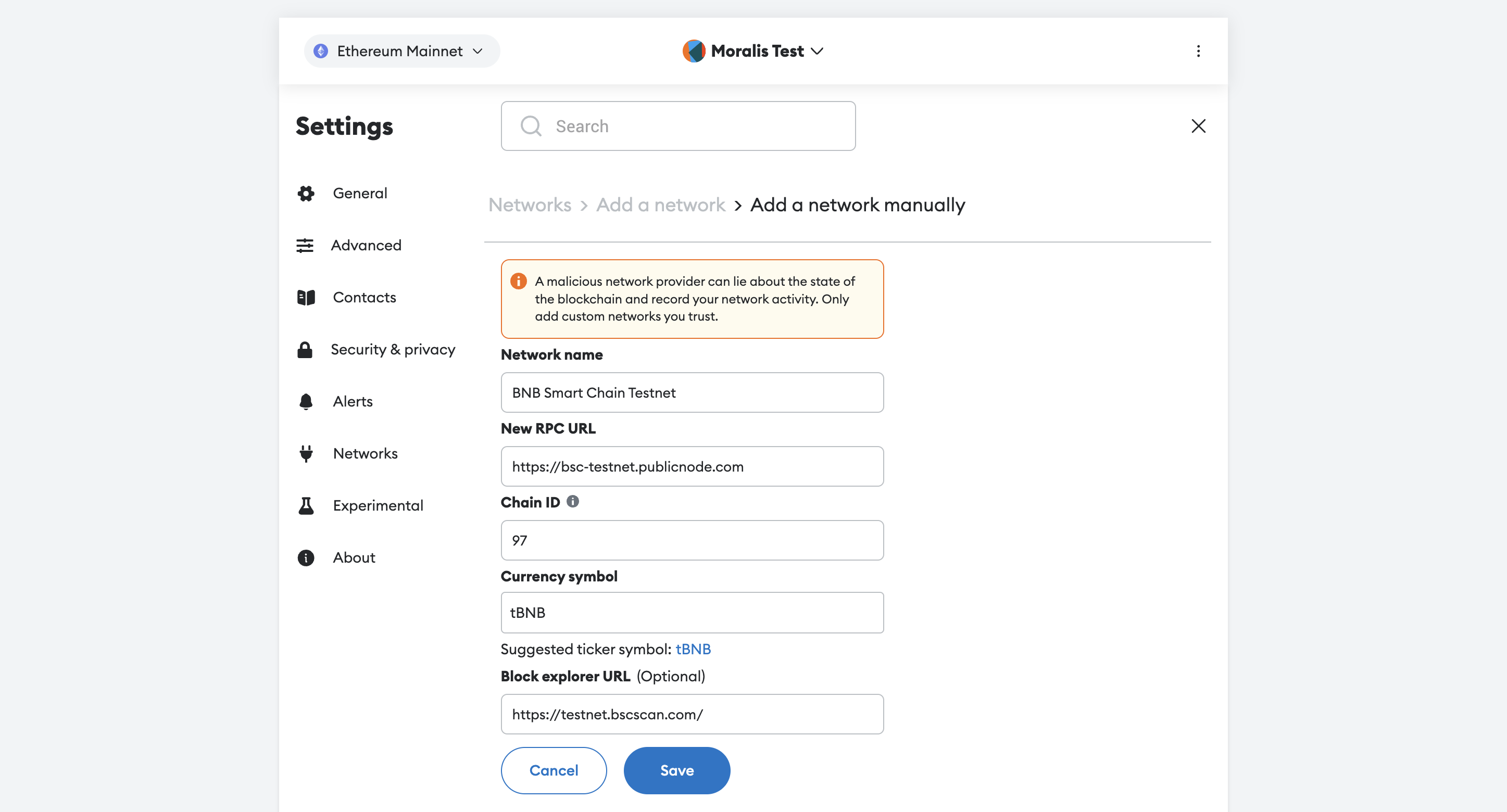
You’ll discover the knowledge you want under:
After hitting “Save”, the community can have been added to your pockets, permitting you to modify to the testnet:
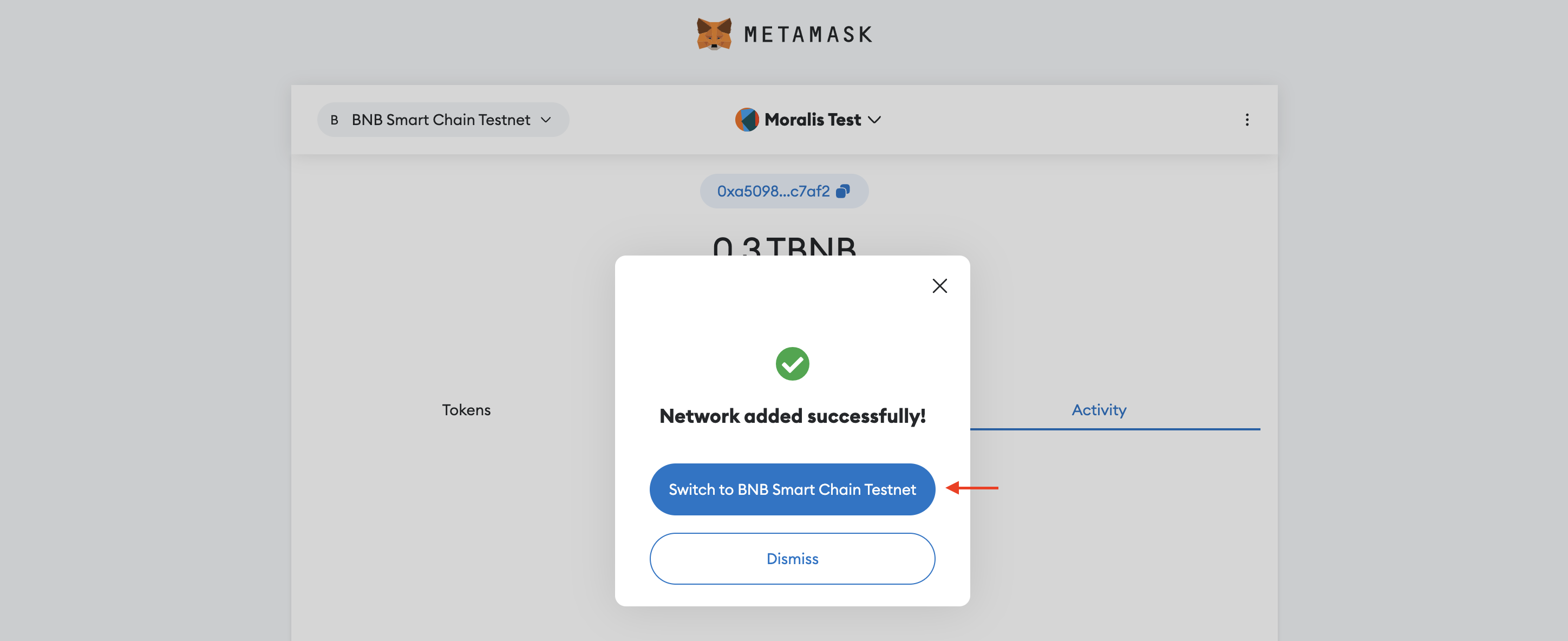
That’s it; you have got now efficiently added the BSC testnet to your MetaMask pockets:
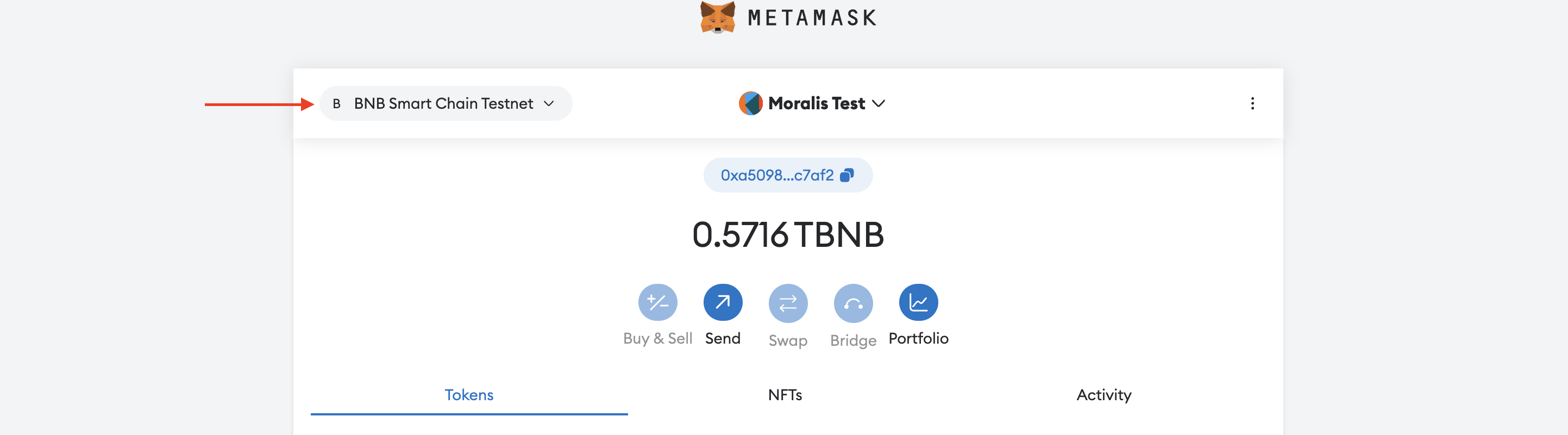
Step 2: Get BSC Testnet Tokens
To get BSC testnet tokens, you want a dependable BNB faucet, and the best strategy to discover one is to make use of Moralis. Merely go to Moralis’ crypto taps web page, scroll down, and click on ”Strive Now” for the BSC various:
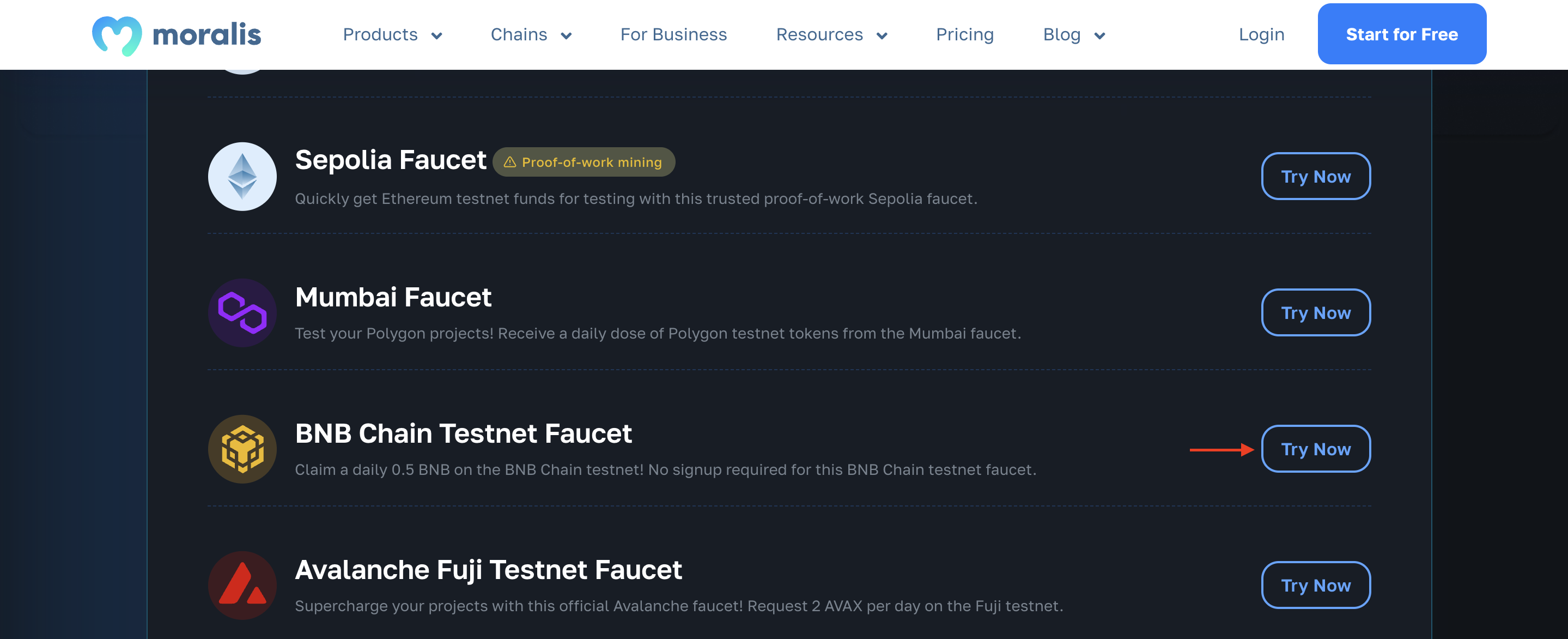
Clicking the button above takes you to the next web page, the place you simply want to stick your MetaMask handle and hit the ”Ship 0.3 BNB” button:
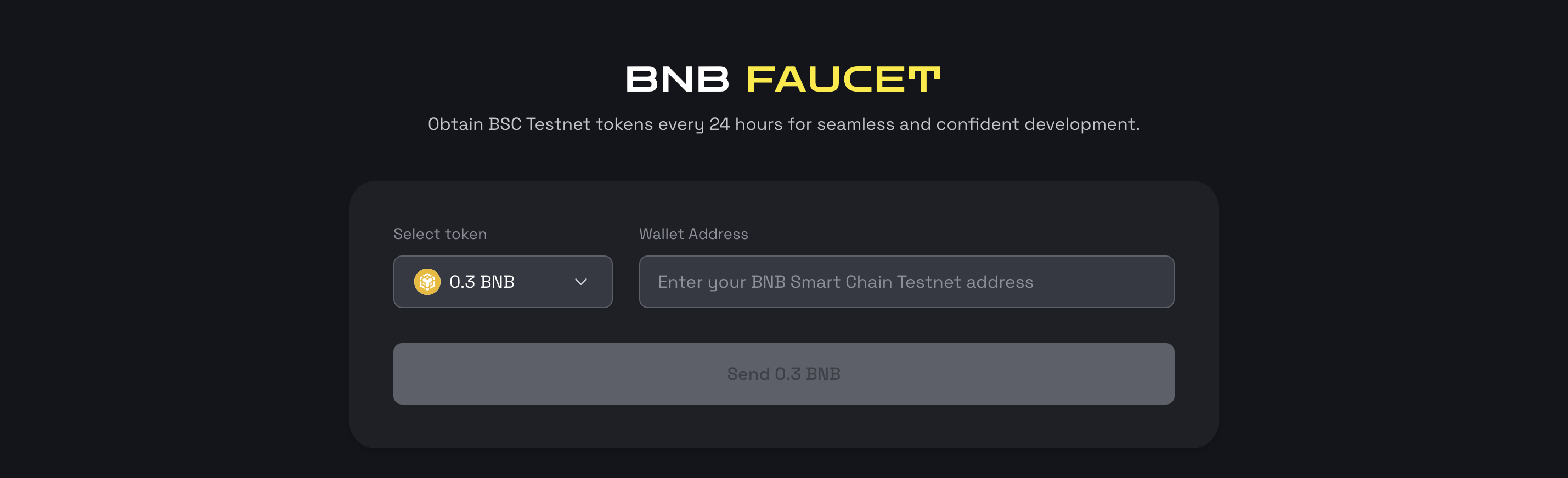
As soon as the transaction is finalized, it’s best to now end up with a further 0.3 BNB testnet tokens added to your MetaMask pockets:
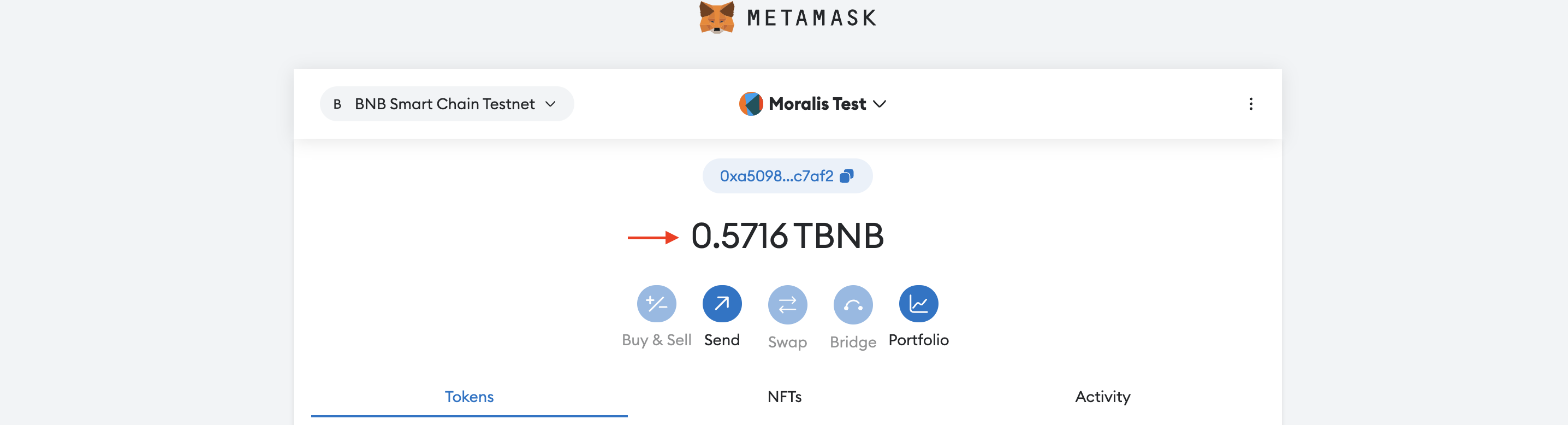
From right here, now you can use these newly acquired tokens to pay for transactions on the BSC testnet!
Step 3: Create Your BSC Token Contract with Remix
Since BSC is EVM-compatible, we will use Solidity and the ERC-20 token commonplace to create BEP-20 tokens. This additional means we will leverage an ERC-20 sensible contract template from OpenZeppelin to make this course of as seamless as doable.
With that stated, let’s begin by launching the Remix IDE and organising a brand new workspace:
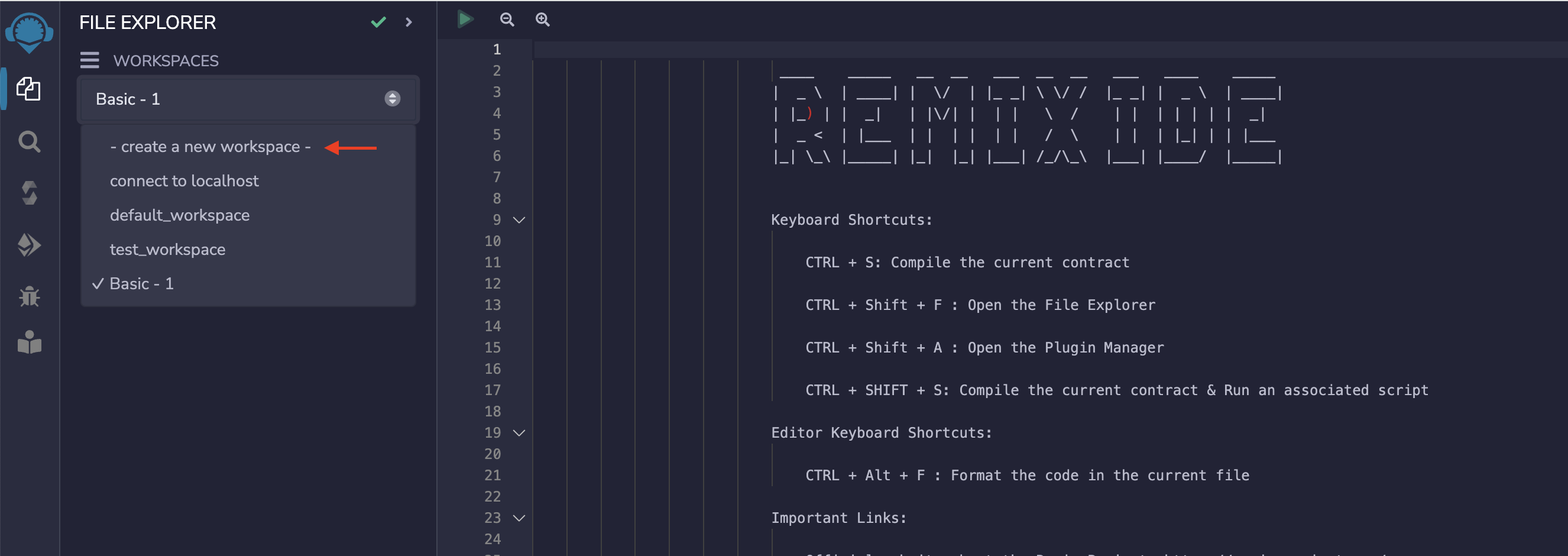
You’ll be able to then create a brand new sensible contract known as one thing like ”BEP20.sol” in your ”contracts” folder:
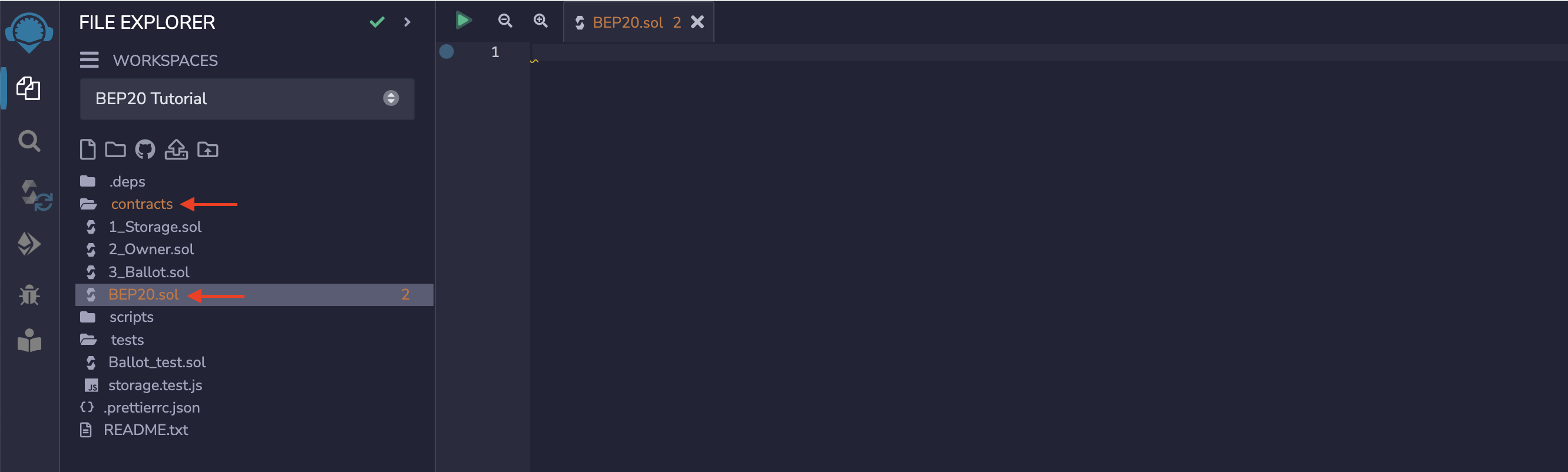
Subsequent, add the next code:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import “@openzeppelin/contracts/token/ERC20/ERC20.sol”;
contract BEP20 is ERC20 {
constructor(uint256 initialSupply) ERC20(“BEP20Test”, “BPT”) {
_mint(msg.sender, initialSupply);
}
}
From right here, let’s now break down the code line by line, ranging from the highest!
We start by specifying the SPDX license:
// SPDX-License-Identifier: MIT
Subsequent, we import the ERC-20 package deal from OpenZeppelin:
import “@openzeppelin/contracts/token/ERC20/ERC20.sol”;
From there, we outline a brand new contract named BEP20, the place we additionally specify that it ought to use the ERC-20 package deal we imported above:
contract BEP20 is ERC20 {
//…
}
We then create a constructor, which is named when the sensible contract is deployed. This constructor takes an initialSupply parameter as an argument, which will probably be used to specify the preliminary provide of our token.
On the identical line, we moreover name the ERC20() perform we imported from OpenZeppelin. This perform takes two parameters as an argument: BEP20Test and BPT. The primary parameter is the title of the token, and the second parameter is its ticker:
constructor(uint256 initialSupply) ERC20(“BEP20Test”, “BPT”) {
//…
}
Lastly, we name the _mint() perform, which is used to create the token. This perform takes two parameters: msg.sender and initialSupply. msg.sender specifies that the tokens will probably be despatched to the handle deploying the contract, and initialSupply units the variety of tokens that will probably be deployed:
_mint(msg.sender, initialSupply);
Step 4: Deploy the Contract
At this level, it’s now time to deploy the sensible contract to the BSC testnet, and to take action, we initially have to compile it. As such, go to the ”Solidity compiler” tab, choose your contract, and hit the ”Compile…” button:
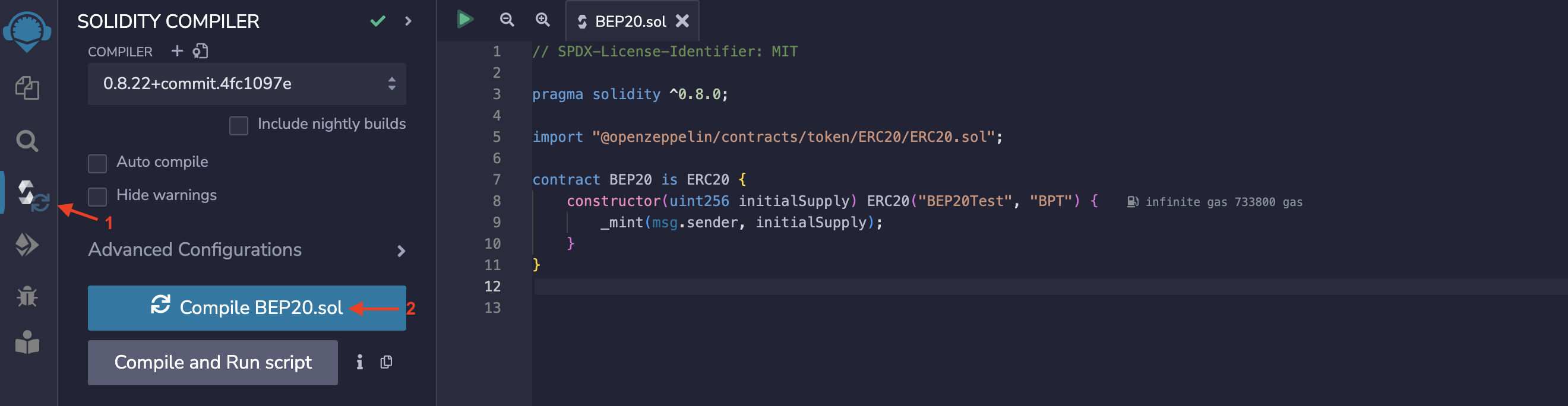
Subsequent, navigate to the ”Deploy & run transactions” tab and choose ”Injected Supplier – …” because the setting:
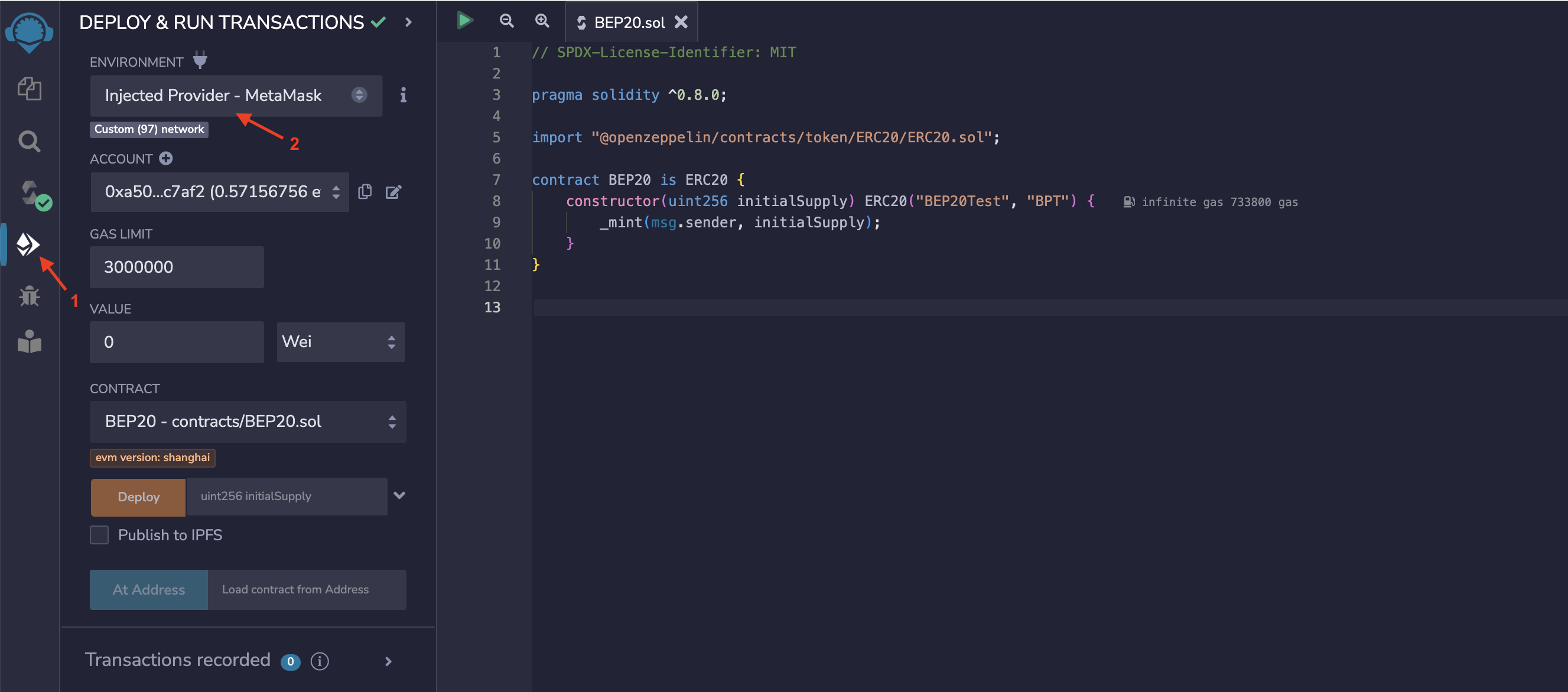
From right here, be certain that you’re deploying the best contract:
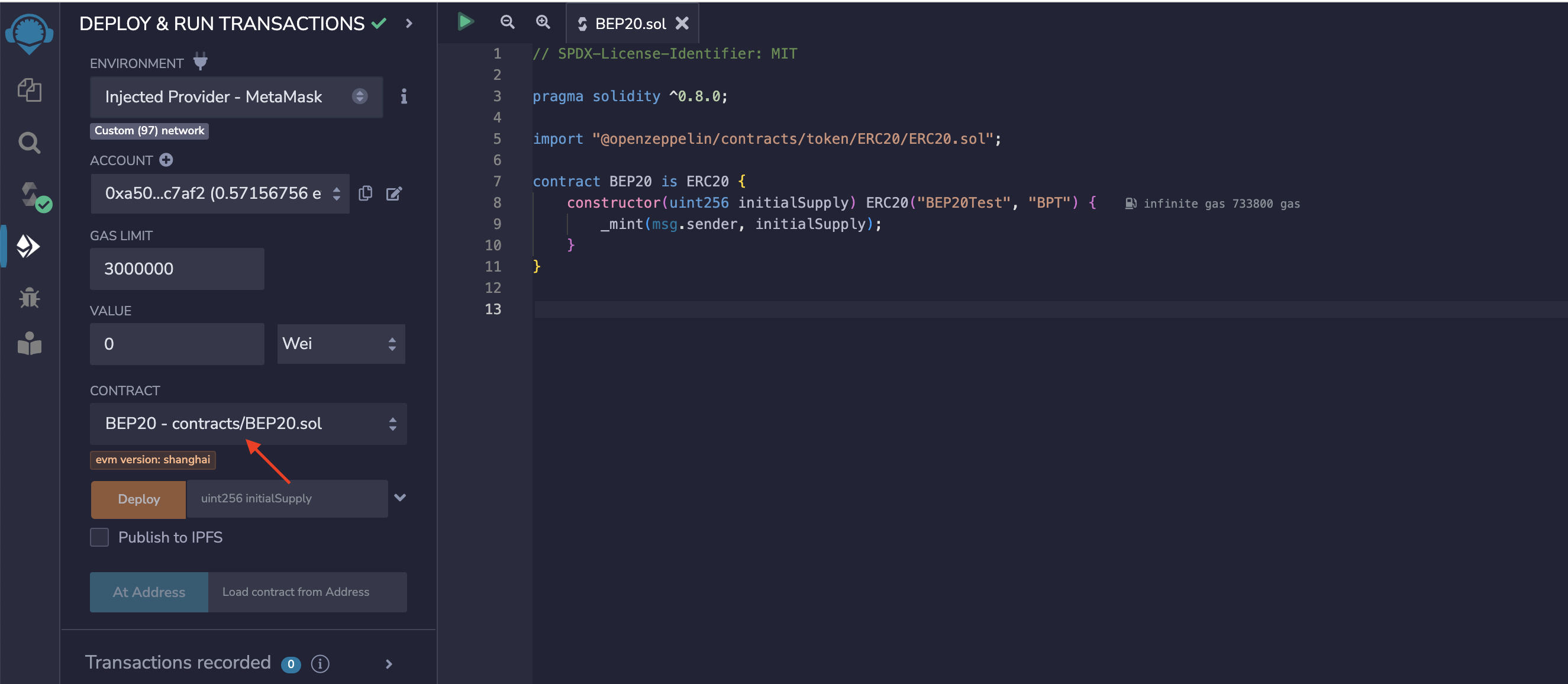
You then have to specify the preliminary token provide that we cross to the contract’s constructor. In our case, we’ll be creating 100 tokens, and because it follows an 18-decimal format, we’ll cross 100000000000000000000 because the parameter:
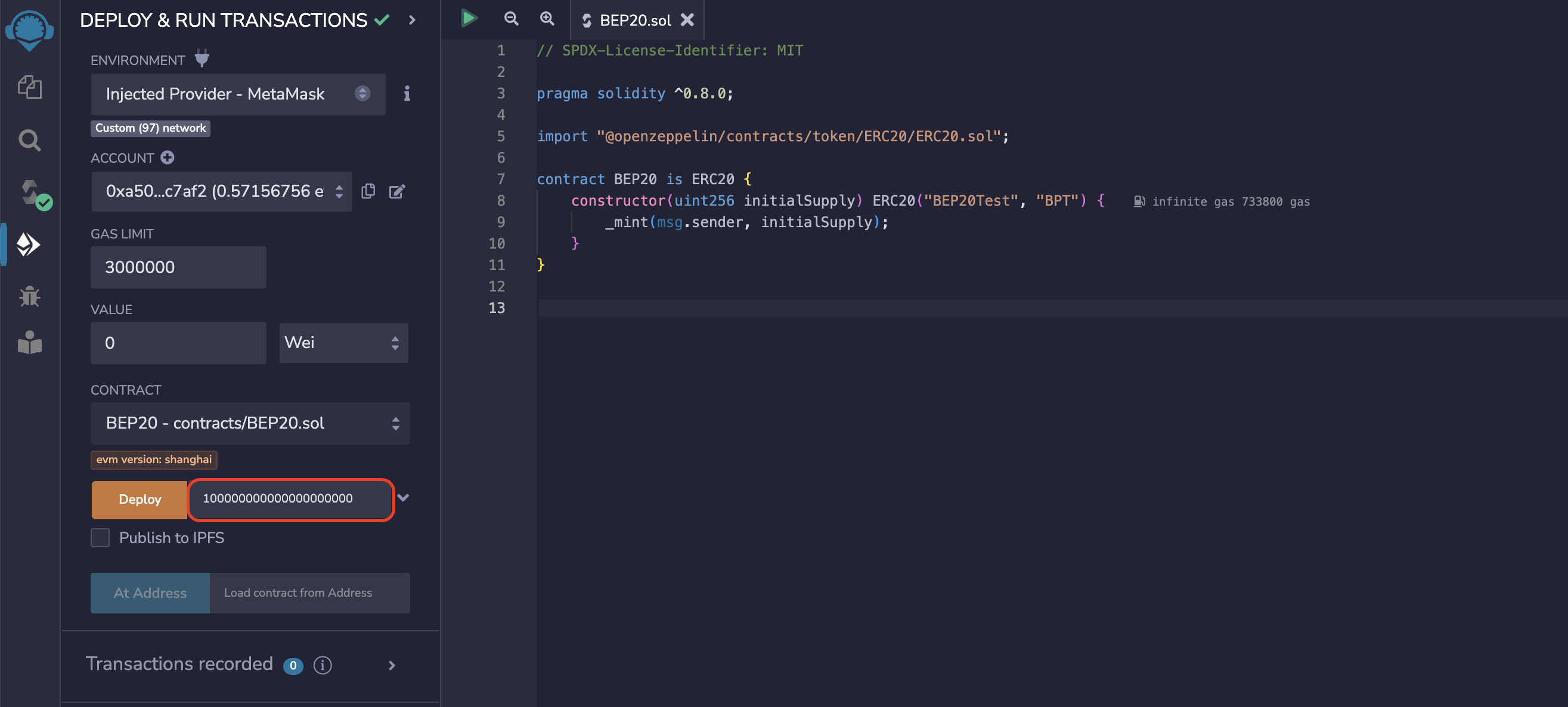
Lastly, all that is still is hitting the ”Deploy” button:
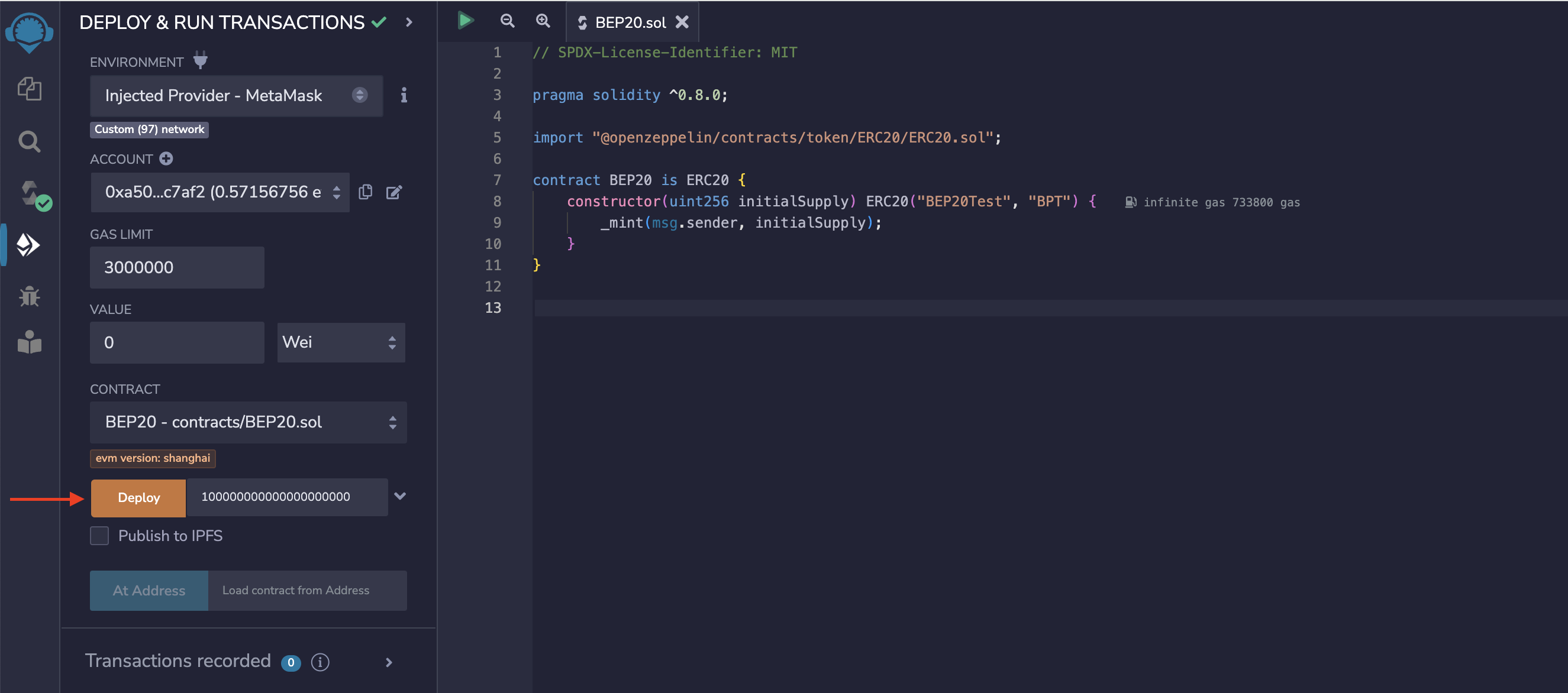
Clicking the ”Deploy” button prompts your MetaMask pockets, as you’ll have to pay for the transaction utilizing the testnet tokens you beforehand acquired:
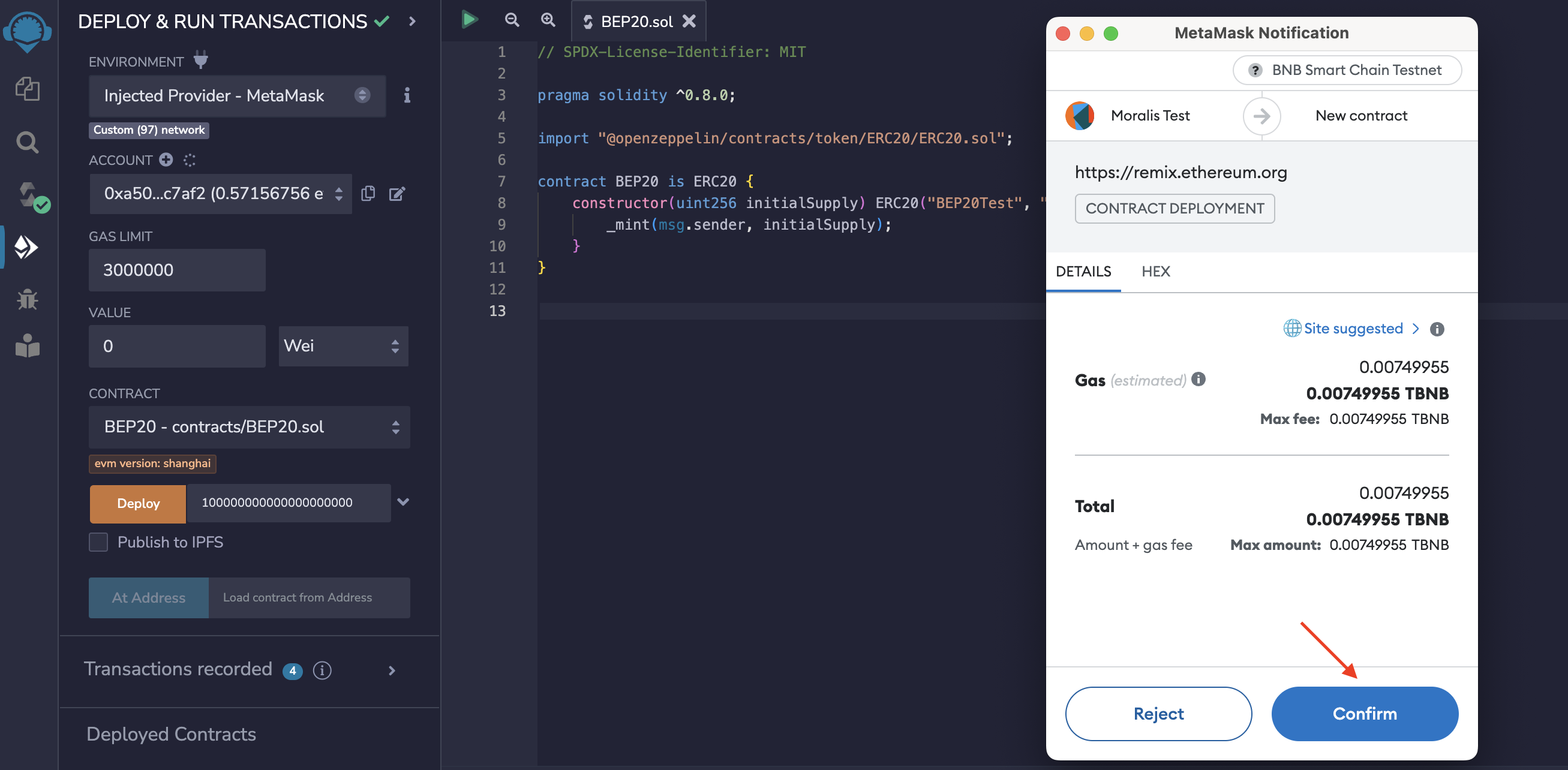
If every little thing went in response to plan, it’s best to now see successful message within the terminal:
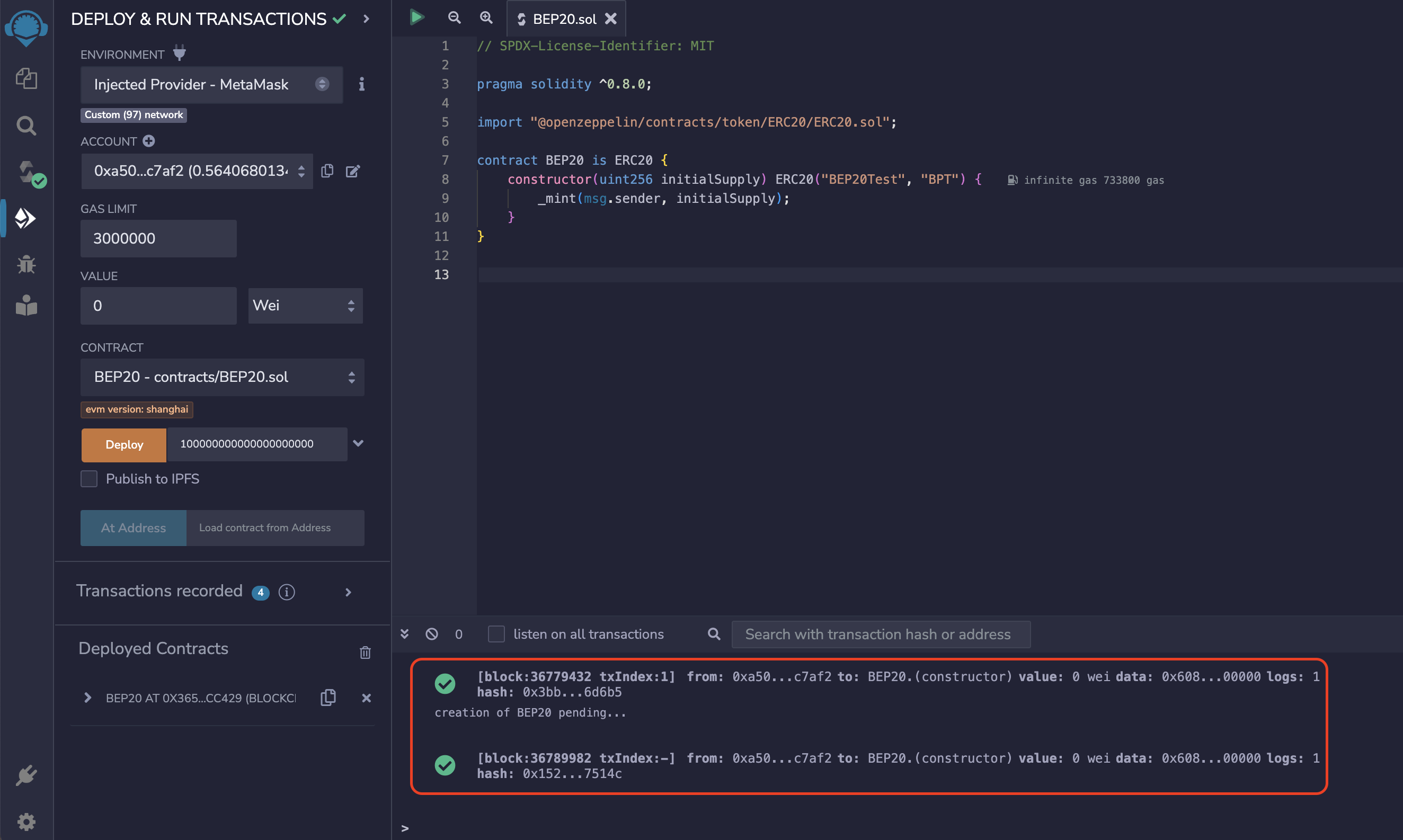
Step 5: Add the Token To MetaMask
So as to add the newly deployed token to your MetaMask pockets, you first want to repeat its contract handle:
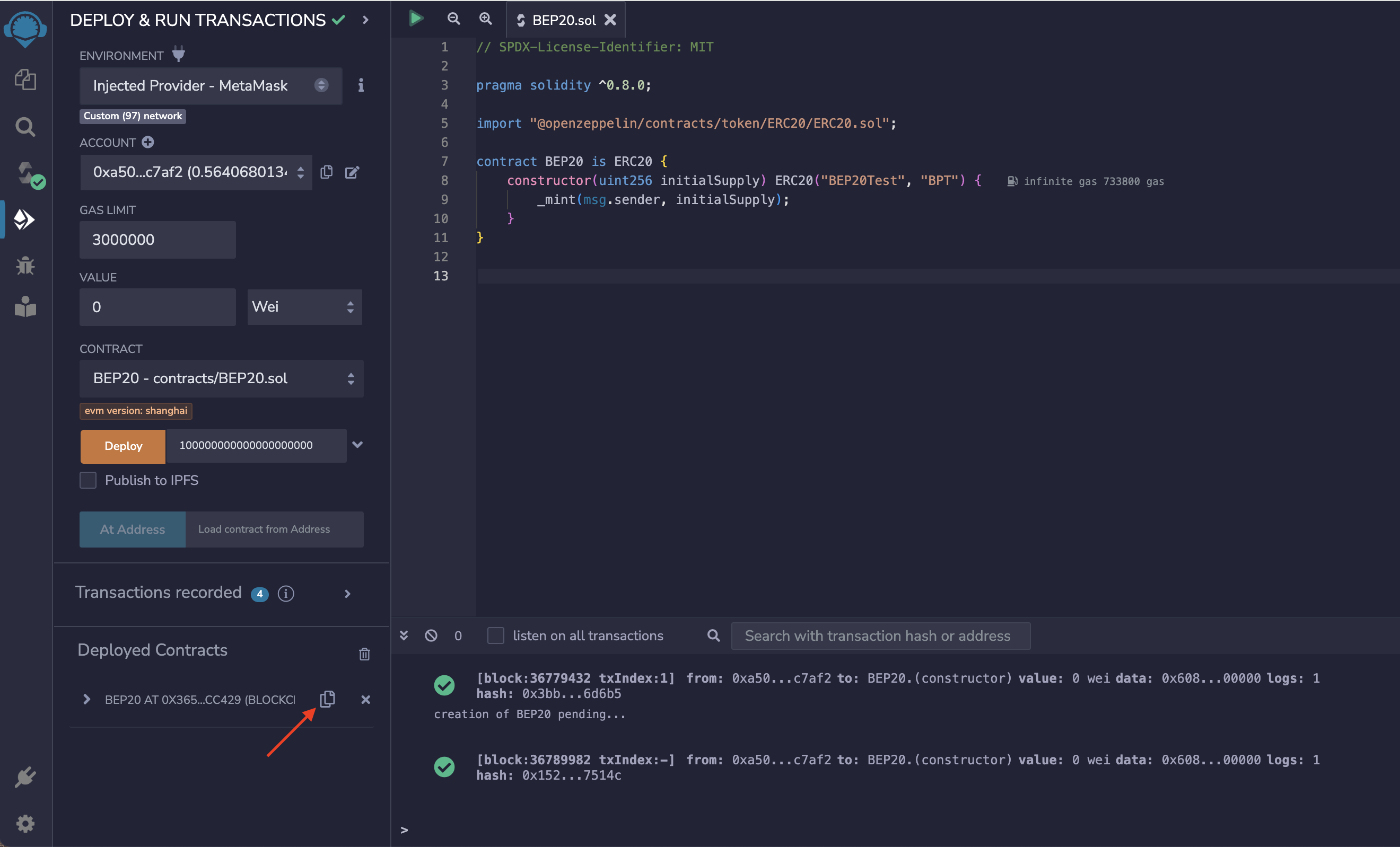
Subsequent, open your MetaMask pockets and click on the ”+ Import tokens” button:
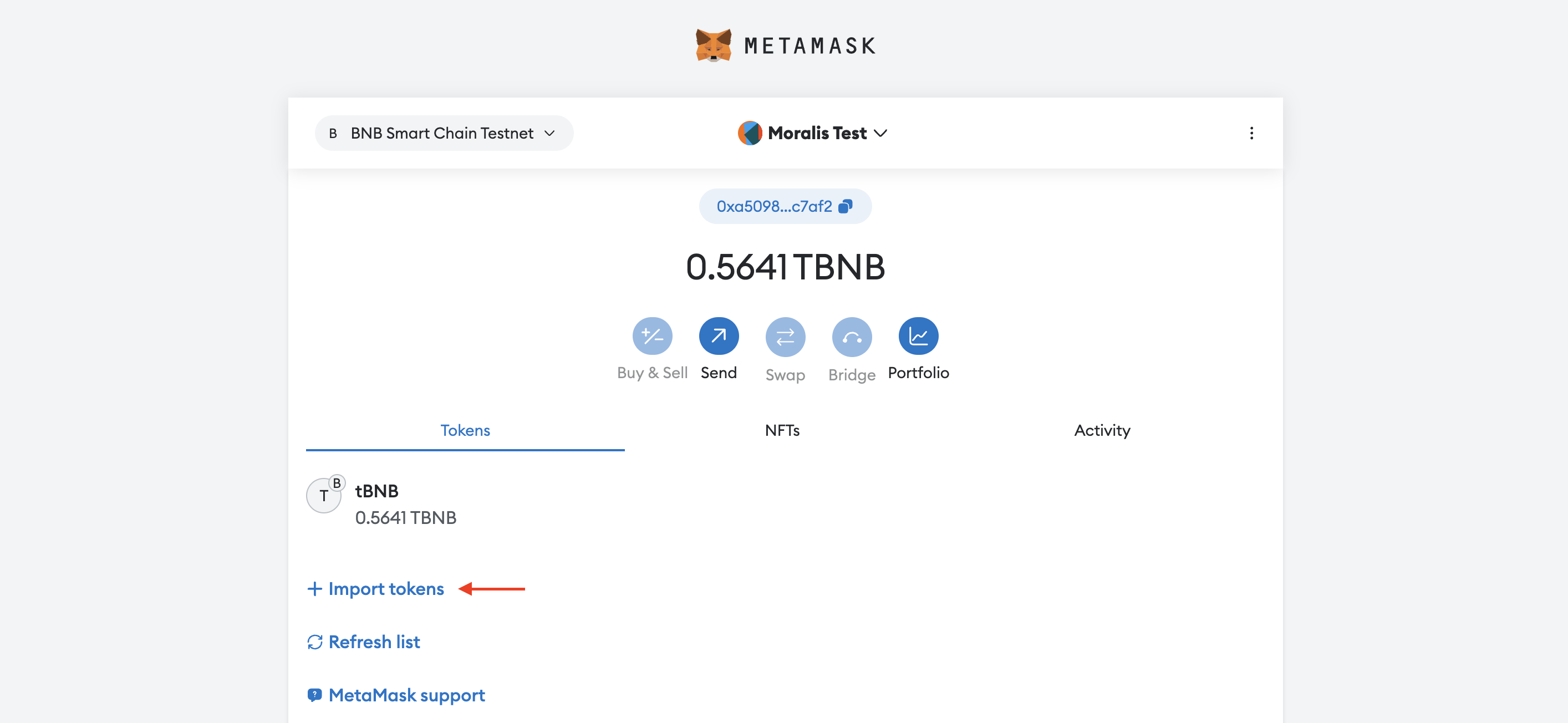
This can open a brand new window the place you merely want to stick the token handle, and the remainder of the knowledge ought to autonomously fill in:
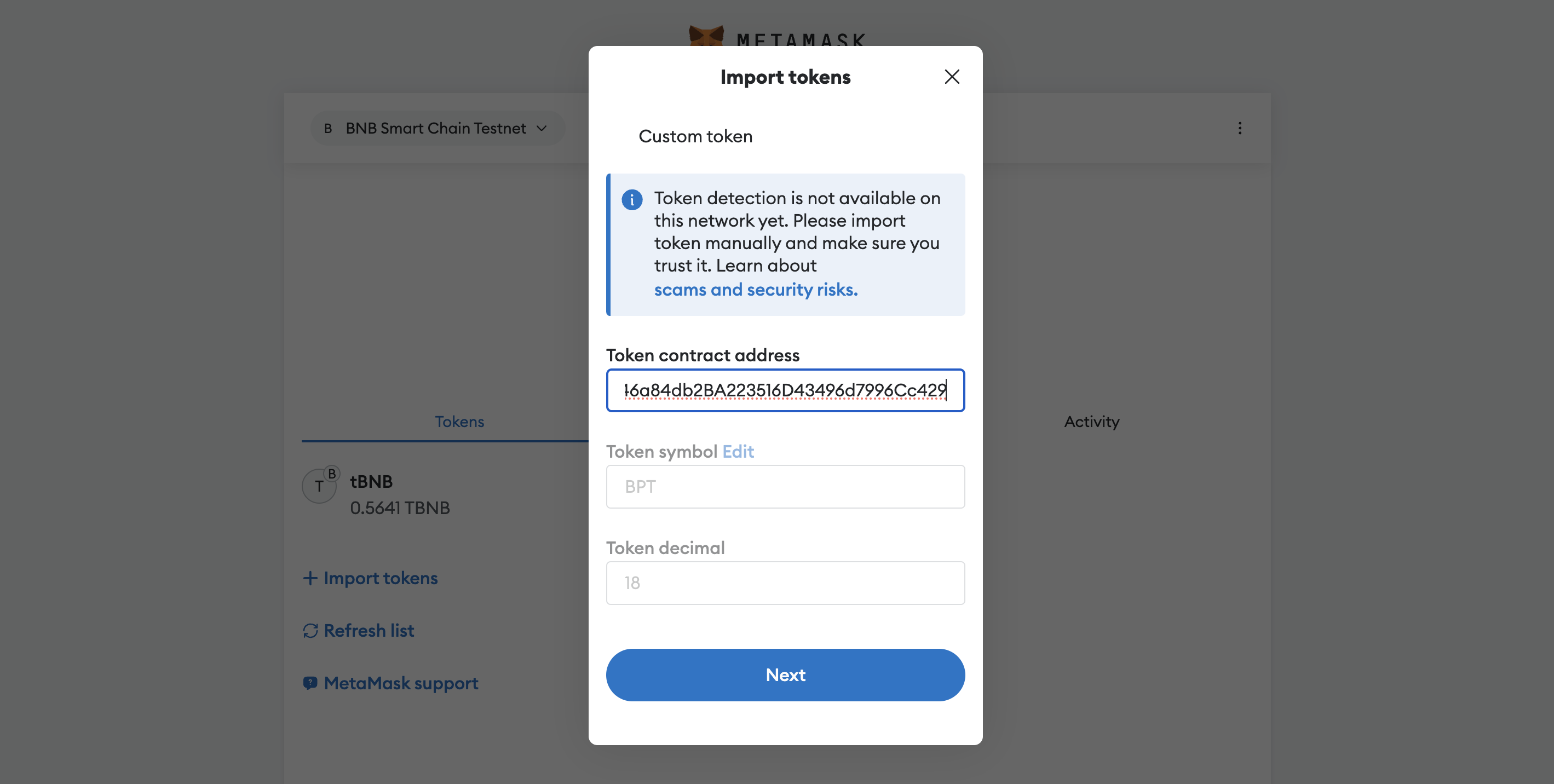
Lastly, click on ”Subsequent” adopted by ”Import”:
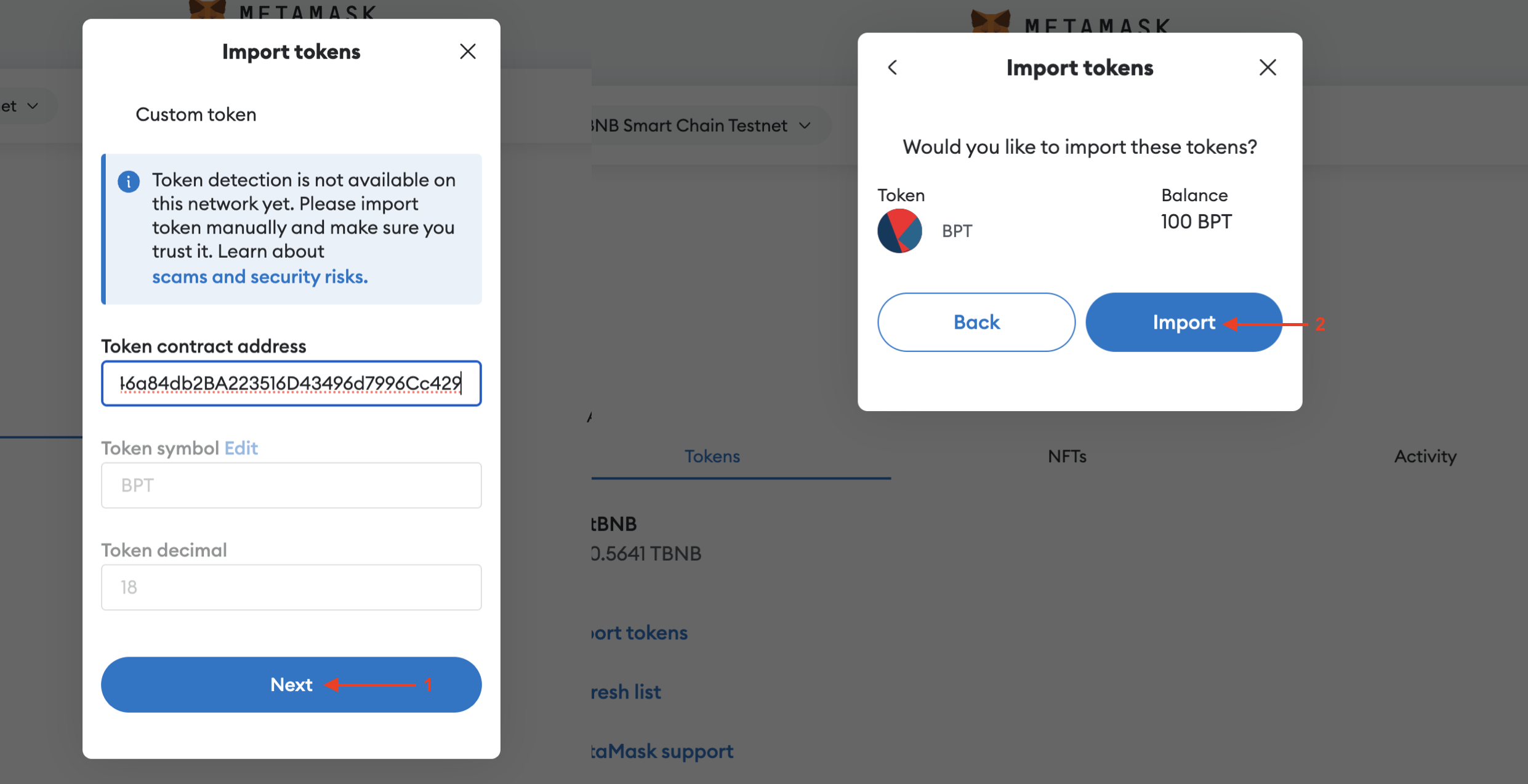
That’s it; it’s best to now see your newly created BSC token in your MetaMask pockets:
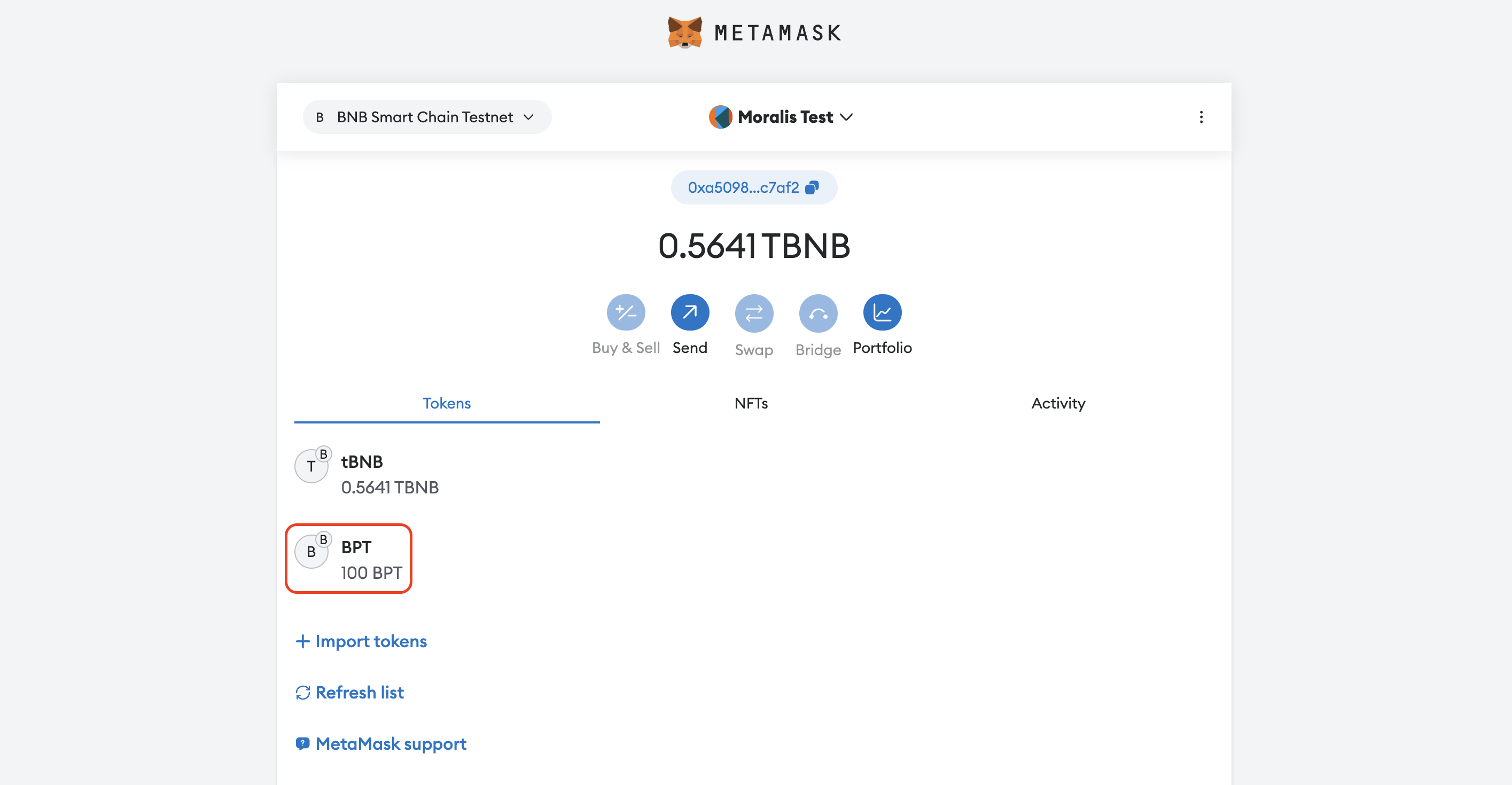
Congratulations! You now know the right way to effortlessly create a token on the BSC testnet. From right here, you may observe just about the identical steps to create a BSC token on the mainnet!
Introducing Moralis – The Business’s Main Web3 API Supplier
Moralis is the {industry}’s main Web3 API supplier, providing a large and dynamic vary of instruments you should use to streamline your growth endeavors. In our suite of premier Web3 APIs, you’ll discover interfaces comparable to our Pockets API, Token API, NFT API, Blockchain API, and lots of others. Consequently, it doesn’t matter when you construct a DEX, Web3 pockets, or every other platform; Moralis has a device for many use circumstances!
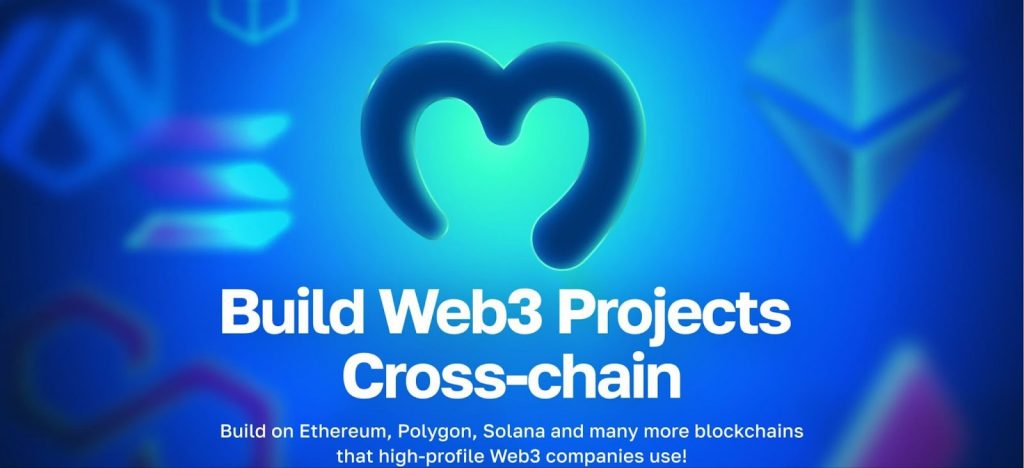
However why do you have to leverage Moralis when constructing dapps?
To reply the above query, let’s discover three advantages of Moralis!
Prime Efficiency: Moralis’ Web3 APIs persistently ship top-tier efficiency. It doesn’t matter whether or not you measure by reliability, velocity, or every other metric; Moralis all the time blows the competitors out of the water. Knowledge Accessibility: With our various set of APIs, you get correct, real-time information with just a few traces of code. As such, when working with Morails, it has by no means been simpler to fetch and combine on-chain information and Web3 performance into your initiatives. Cross-Chain Compatability: Our Web3 APIs are chain-agnostic, supporting all the largest blockchains, together with networks like Ethereum, Polygon, Arbitrum, Solana, and, after all, BNB Good Chain, making our suite of instruments stand out as the final word BSC API.
To study extra about our industry-leading growth instruments, try our Web3 API web page!
Additionally, keep in mind that you would be able to join with Moralis without cost. So, take this chance, and you can begin constructing Web3 initiatives quicker and extra effectively at present!
Abstract: The best way to Create a Token on BSC with Remix
In at present’s article, we kicked issues off by exploring the ins and outs of BSC tokens. In doing so, we discovered that they’re belongings constructed on BNB Good Chain adhering to the BEP-20 token commonplace.
From there, we then explored Remix and MetaMask. Remix is a outstanding IDE for writing, testing, debugging, compiling, and deploying EVM-compatible sensible contracts. In the meantime, MetaMask is a Web3 pockets for storing and managing digital belongings, together with interacting with dapps and sensible contracts.
Subsequent, we confirmed you the right way to create a token on BSC with Remix in 5 simple steps:
Add the BSC Testnet to MetaMaskGet BSC Testnet TokensCreate Your BSC Token Contract with RemixDeploy Your ContractAdd the Token To MetaMask
As such, when you’ve got adopted alongside this far, you now know the right way to create a BSC token with Remix!
For those who appreciated this tutorial on the right way to create a BSC token, take into account trying out extra Moralis content material. As an illustration, learn our contract ABI information or discover ways to record all of the cash in an ETH handle.
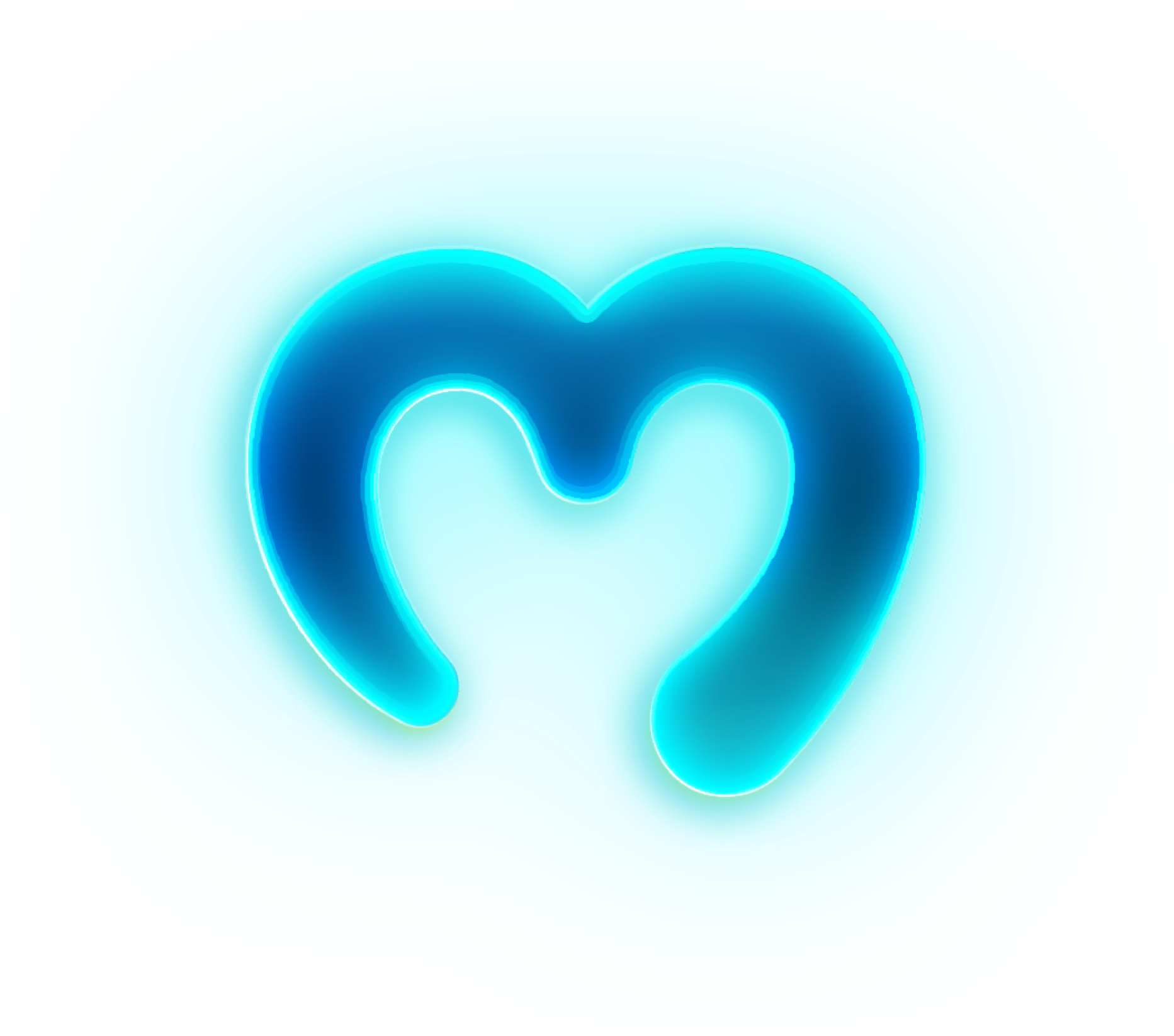
Moreover, for these excited to embark on their journey in Web3 growth, you’ll want to join with Moralis. Signing up is free, and also you’ll acquire prompt entry to all our premier Web3 APIs!